js获取最近七天的开始结束时间
时间: 2024-09-30 07:07:32 浏览: 44
JavaScript 获取最近七天的开始和结束时间,你可以使用`Date`对象配合`setTime()`方法来实现。下面是一个简单的示例:
```javascript
// 获取当前日期
const currentDate = new Date();
// 减去7天的时间差
const sevenDaysAgo = currentDate.setDate(currentDate.getDate() - 7);
// 设置新的开始日期
const startDate = new Date(sevenDaysAgo);
startDate.setHours(0, 0, 0, 0); // 设置为每天的0点
// 设置新的结束日期
const endDate = new Date(sevenDaysAgo);
endDate.setHours(23, 59, 59, 999); // 设置为每天的23点59分59秒(考虑到毫秒)
startDate.toString(); // 输出开始日期,例如:"Mon Jun 06 2022 00:00:00 GMT+0800 (中国标准时间)"
endDate.toString(); // 输出结束日期,例如:"Sun Jun 12 2022 23:59:59 GMT+0800 (中国标准时间)"
相关问题
js获取当前周及当前周的开始结束时间
在JavaScript中,获取当前周以及其开始和结束的时间,可以利用`Date`对象以及一些额外的计算。下面是一个示例:
```javascript
// 获取当前日期
let currentDate = new Date();
// 转换为周日作为周的开始 (0 表示周日)
currentDate.setHours(0, 0, 0, 0);
currentDate.setDate(currentDate.getDate() - currentDate.getDay());
// 当前周的开始时间
let startOfWeek = currentDate;
// 计算当前周的结束时间,因为JavaScript的Date对象不会自动包含周末,所以需要加一天
let endOfWeek = new Date(startOfWeek);
endOfWeek.setDate(endOfWeek.getDate() + 6);
console.log('本周开始:', startOfWeek);
console.log('本周结束:', endOfWeek);
```
在这个例子中,我们首先获取了当前日期,然后将其调整到周日(因为默认的周日是一周的开始)。接着,我们创建了一个新的`Date`实例来表示周的结束,通常是在当前周的基础上加上六天(因为一周有七天)。
注意,这个计算假设每周从周日开始,并且周六是最后一天。如果您的需求不同(比如某些地方习惯周一作为一周的第一天),则需要相应地调整。
el-date-picker只能选择从当前时间开始七天后的日期
`el-date-picker`是一个基于Element UI的日期选择组件,在Vue.js中常用于处理日期相关的交互。如果你想要限制用户只能选择从当前时间开始七天后的日期,你可以通过设置它的`default-value`属性或者`picker-options`对象来实现。这里有一个简单的例子:
```html
<template>
<el-date-picker
v-model="startDate"
type="daterange"
range-separator="至"
start-placeholder="开始日期"
end-placeholder="结束日期"
:picker-options="{
disabledDate (current) {
return current && current < moment().add(7, 'days');
}
}"
></el-date-picker>
</template>
<script>
import { ElDatePicker } from "element-ui";
import moment from "moment";
export default {
components: {
ElDatePicker
},
data() {
return {
startDate: null,
};
},
methods: {
// 如果需要获取到用户输入的实际日期范围,可以在这里添加事件处理器
},
};
</script>
```
在这个示例中,`disabledDate`回调函数会阻止用户选择小于当前日期加上七天的日期。当用户点击日期选择器时,如果所选日期不符合这个条件,它将变为不可选。
阅读全文
相关推荐




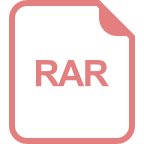





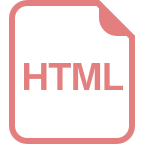




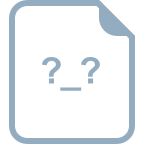
