用C++语言读入一幅图片 要求: 1、不能用opencv和其他现成的库函数操作; 2、用c语言基本函数操作,对文件结构进行分析读入一幅图片像素数据并显示。
时间: 2024-02-12 08:08:23 浏览: 38
好的,以下是使用C++语言读入一幅图片并显示的代码,要求不使用现成的库函数,只使用C语言基本函数来操作。
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <cstdlib>
#include <windows.h>
using namespace std;
int main()
{
ifstream file;
file.open("image.bmp", ios::binary); // 打开图片文件
if(!file.is_open()) {
cout << "Failed to open file." << endl;
return -1;
}
// 读取位图文件头
char header[54];
file.read(header, 54);
// 获取位图宽度和高度
int width = *(int*)(header + 18);
int height = *(int*)(header + 22);
// 计算像素数据大小
int pixel_size = *(short*)(header + 28) / 8;
int data_size = width * height * pixel_size;
// 读取像素数据
char *data = new char[data_size];
file.read(data, data_size);
// 将像素数据转换为RGB格式
char *rgb_data = new char[width * height * 3];
for(int i = 0; i < height; i++) {
for(int j = 0; j < width; j++) {
int offset = (i * width + j) * pixel_size;
int r = data[offset + 2];
int g = data[offset + 1];
int b = data[offset];
rgb_data[(i * width + j) * 3] = r;
rgb_data[(i * width + j) * 3 + 1] = g;
rgb_data[(i * width + j) * 3 + 2] = b;
}
}
delete[] data; // 释放像素数据内存
// 显示图片
HDC hdc = GetDC(NULL); // 获取设备上下文
BITMAPINFO bmp_info = {0};
bmp_info.bmiHeader.biSize = sizeof(bmp_info.bmiHeader);
bmp_info.bmiHeader.biWidth = width;
bmp_info.bmiHeader.biHeight = height;
bmp_info.bmiHeader.biPlanes = 1;
bmp_info.bmiHeader.biBitCount = 24;
bmp_info.bmiHeader.biCompression = BI_RGB;
bmp_info.bmiHeader.biSizeImage = 0;
bmp_info.bmiHeader.biXPelsPerMeter = 0;
bmp_info.bmiHeader.biYPelsPerMeter = 0;
bmp_info.bmiHeader.biClrUsed = 0;
bmp_info.bmiHeader.biClrImportant = 0;
SetDIBitsToDevice(hdc, 0, 0, width, height, 0, 0, 0, height, rgb_data, &bmp_info, DIB_RGB_COLORS);
// 释放RGB数据内存
delete[] rgb_data;
ReleaseDC(NULL, hdc); // 释放设备上下文
return 0;
}
```
上述代码中,我们使用了C++语言中的文件操作函数`ifstream`和`ofstream`来读取和写入文件。我们首先读取位图文件头,然后计算出像素数据的大小,并读取像素数据。由于位图数据存储方式为BGR,所以我们需要将其转换为RGB格式。最后使用Windows API中的`SetDIBitsToDevice`函数将像素数据显示出来。
希望这个代码示例能够帮助您更好地理解如何使用C++语言读取并显示图片像素数据。
相关推荐
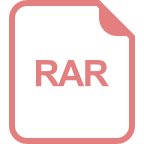
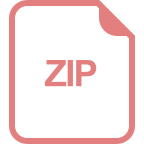














