用c++语言写一个反四子棋的bot程序
时间: 2023-09-14 08:08:14 浏览: 176
以下是一个简单的反四子棋 Bot 程序,仅供参考:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
const int SIZE = 15; // 棋盘大小
const int WIN = 5; // 胜利所需棋子数
const int MAX_DEPTH = 4; // 最大搜索深度
const int INF = 0x3f3f3f3f; // 无穷大
int board[SIZE][SIZE]; // 棋盘
int weights[WIN + 1] = {0, 1, 10, 100, 1000, 10000}; // 棋型分值
int player = 1; // 玩家执子
int bot = -1; // 机器人执子
// 判断是否在棋盘内
bool inBoard(int x, int y) {
return x >= 0 && x < SIZE && y >= 0 && y < SIZE;
}
// 判断是否是空位
bool isEmpty(int x, int y) {
return inBoard(x, y) && board[x][y] == 0;
}
// 判断是否胜利
bool isWin(int x, int y, int player) {
int dx[4] = {1, 0, 1, -1};
int dy[4] = {0, 1, 1, 1};
for (int k = 0; k < 4; k++) {
int cnt = 0;
for (int i = x, j = y; inBoard(i, j) && board[i][j] == player; i += dx[k], j += dy[k]) {
cnt++;
}
for (int i = x - dx[k], j = y - dy[k]; inBoard(i, j) && board[i][j] == player; i -= dx[k], j -= dy[k]) {
cnt++;
}
if (cnt >= WIN) {
return true;
}
}
return false;
}
// 评估当前局面的优劣
int evaluate(int player) {
int score = 0;
for (int k = 1; k <= WIN; k++) {
int cnt = 0;
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (board[i][j] == player) {
cnt++;
} else if (board[i][j] == -player) {
cnt = 0;
break;
}
}
if (cnt == k) {
score += weights[k];
}
}
}
return score;
}
// 极大极小值搜索
int alphaBeta(int depth, int alpha, int beta, int player) {
if (depth == 0) {
return evaluate(bot) - evaluate(player);
}
int bestScore = -INF;
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (isEmpty(i, j)) {
board[i][j] = bot;
int score = -alphaBeta(depth - 1, -beta, -alpha, -player);
board[i][j] = 0;
bestScore = max(bestScore, score);
alpha = max(alpha, score);
if (alpha >= beta) {
return bestScore;
}
}
}
}
return bestScore;
}
// 机器人下棋
void botMove() {
int bestScore = -INF;
int bestX = -1, bestY = -1;
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (isEmpty(i, j)) {
board[i][j] = bot;
int score = -alphaBeta(MAX_DEPTH, -INF, INF, player);
board[i][j] = 0;
if (score > bestScore) {
bestScore = score;
bestX = i;
bestY = j;
}
}
}
}
board[bestX][bestY] = bot;
cout << "Bot move: (" << bestX << ", " << bestY << ")" << endl;
}
// 玩家下棋
void playerMove() {
int x, y;
cout << "Player move: ";
cin >> x >> y;
while (!isEmpty(x, y)) {
cout << "Invalid move, please try again: ";
cin >> x >> y;
}
board[x][y] = player;
}
// 打印棋盘
void printBoard() {
cout << " ";
for (int j = 0; j < SIZE; j++) {
cout << j << " ";
}
cout << endl;
for (int i = 0; i < SIZE; i++) {
cout << i << " ";
for (int j = 0; j < SIZE; j++) {
if (board[i][j] == player) {
cout << "o ";
} else if (board[i][j] == bot) {
cout << "x ";
} else {
cout << "+ ";
}
}
cout << endl;
}
}
int main() {
// 初始化棋盘
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
board[i][j] = 0;
}
}
// 选择执子
cout << "Choose your side (o/x): ";
char side;
cin >> side;
if (side == 'o') {
player = 1;
bot = -1;
} else {
player = -1;
bot = 1;
botMove();
}
// 开始游戏
while (true) {
printBoard();
if (isWin(board[SIZE / 2][SIZE / 2], SIZE / 2, player)) {
cout << "You win!" << endl;
break;
}
if (isWin(board[SIZE / 2][SIZE / 2], SIZE / 2, bot)) {
cout << "Bot win!" << endl;
break;
}
if (player == 1) {
playerMove();
} else {
botMove();
}
swap(player, bot);
}
return 0;
}
```
以上程序使用了极大极小值搜索算法,并实现了简单的评估函数和搜索剪枝策略。在实现过程中需要注意以下几个问题:
- 如何表示棋盘和棋子:使用二维数组来表示棋盘,用 1 表示玩家的棋子,-1 表示机器人的棋子,0 表示空位。
- 如何进行搜索:使用递归实现极大极小值搜索,设置搜索深度和α-β剪枝策略。
- 如何进行下棋:使用键盘输入方式进行下棋,同时需要更新棋盘状态和当前局面评估值。
- 如何判断胜负:使用判断是否连成五子来判断胜负。
最后,需要提醒的是,以上程序仅供参考,具体实现过程中需要根据自己的情况进行调整和优化。
阅读全文
相关推荐
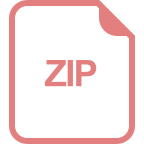
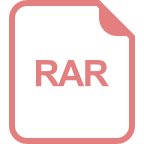
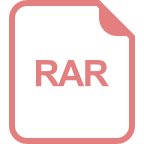
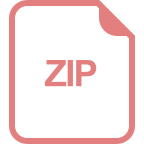
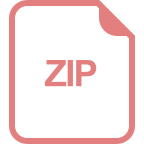
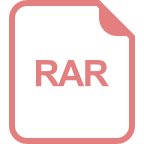
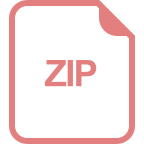
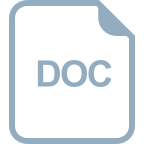
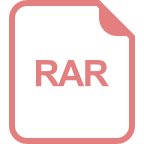
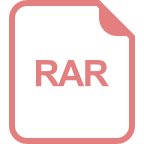
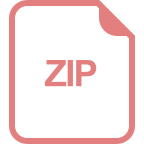
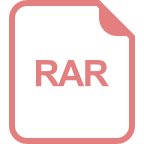