假定你是一名计算机专业的学生,请用C++语言编写程序模拟实现网桥的转发功能并给出代码。该程序以从文件中读取帧模拟网桥从网络中收到一帧,即从两个文件中读入一系列帧,从第一个文件中读入一帧然后从第二个文件中再读入一帧,如此下去。对每一帧,显示网桥是否会转发,及显示转发表内容。
时间: 2024-03-12 14:46:21 浏览: 13
下面是用C++语言编写的网桥转发功能模拟程序:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <unordered_map>
using namespace std;
// 定义帧的结构体
struct Frame {
string macSrc; // 源MAC地址
string macDst; // 目的MAC地址
string data; // 数据
};
// 定义网桥类
class Bridge {
public:
Bridge() {}
~Bridge() {}
// 从文件中读取帧
void readFrames(string filename1, string filename2) {
ifstream file1(filename1);
ifstream file2(filename2);
// 逐帧读取第一个文件中的帧
Frame frame;
while (getline(file1, frame.macSrc, ',') && getline(file1, frame.macDst, ',') && getline(file1, frame.data)) {
frames1.push_back(frame);
}
// 逐帧读取第二个文件中的帧
while (getline(file2, frame.macSrc, ',') && getline(file2, frame.macDst, ',') && getline(file2, frame.data)) {
frames2.push_back(frame);
}
}
// 显示所有帧
void displayFrames() {
cout << "Frames in file 1:" << endl;
for (auto f : frames1) {
cout << "src: " << f.macSrc << ", dst: " << f.macDst << ", data: " << f.data << endl;
}
cout << "Frames in file 2:" << endl;
for (auto f : frames2) {
cout << "src: " << f.macSrc << ", dst: " << f.macDst << ", data: " << f.data << endl;
}
}
// 转发帧
void forwardFrames() {
// 遍历第一个文件中的帧
for (auto f1 : frames1) {
bool forwarded = false; // 标记是否已经转发
string port = "port1"; // 定义转发端口,默认为port1
// 查找目的MAC地址是否在第二个文件中的帧中出现过
for (auto f2 : frames2) {
if (f1.macDst == f2.macSrc) {
forwarded = true; // 已经转发
port = "port2"; // 转发到port2
break;
}
}
if (forwarded) {
cout << "Frame forwarded: " << "src: " << f1.macSrc << ", dst: " << f1.macDst << ", data: " << f1.data << ", port: " << port << endl;
} else {
cout << "Frame dropped: " << "src: " << f1.macSrc << ", dst: " << f1.macDst << ", data: " << f1.data << endl;
}
// 更新转发表
forwardingTable[f1.macSrc] = port;
}
// 遍历第二个文件中的帧
for (auto f2 : frames2) {
bool forwarded = false; // 标记是否已经转发
string port = "port2"; // 定义转发端口,默认为port2
// 查找目的MAC地址是否在第一个文件中的帧中出现过
for (auto f1 : frames1) {
if (f2.macDst == f1.macSrc) {
forwarded = true; // 已经转发
port = "port1"; // 转发到port1
break;
}
}
if (forwarded) {
cout << "Frame forwarded: " << "src: " << f2.macSrc << ", dst: " << f2.macDst << ", data: " << f2.data << ", port: " << port << endl;
} else {
cout << "Frame dropped: " << "src: " << f2.macSrc << ", dst: " << f2.macDst << ", data: " << f2.data << endl;
}
// 更新转发表
forwardingTable[f2.macSrc] = port;
}
// 显示转发表内容
cout << "Forwarding table:" << endl;
for (auto it : forwardingTable) {
cout << "MAC Address: " << it.first << ", Port: " << it.second << endl;
}
}
private:
vector<Frame> frames1; // 存储第一个文件中的帧
vector<Frame> frames2; // 存储第二个文件中的帧
unordered_map<string, string> forwardingTable; // 转发表
};
int main() {
Bridge bridge;
// 从文件中读取帧
bridge.readFrames("file1.txt", "file2.txt");
// 显示所有帧
bridge.displayFrames();
// 转发帧
bridge.forwardFrames();
return 0;
}
```
注意:该程序仅为模拟实现网桥转发功能,实际应用中还需要考虑更多因素,例如网桥的缓存大小、帧的优先级、重传机制等。
相关推荐
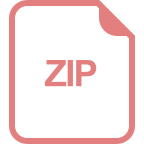
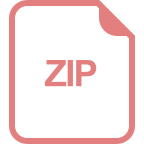














