用c++利用栈的基本操作实现将任意一个十进制整数转化为R进制整数
时间: 2024-05-05 21:15:12 浏览: 102
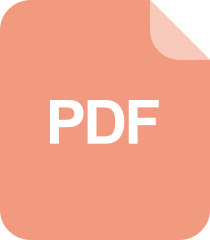
C++实现十六进制字符串转换为十进制整数的方法
以下是用 C 语言实现将任意一个十进制整数转化为 R 进制整数的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct Stack {
int top;
int data[MAX_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
if (s->top == -1) {
return 1;
}
return 0;
}
int is_full(Stack *s) {
if (s->top == MAX_SIZE - 1) {
return 1;
}
return 0;
}
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack overflow\n");
exit(1);
}
s->top++;
s->data[s->top] = x;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(1);
}
int x = s->data[s->top];
s->top--;
return x;
}
int main() {
int n, r;
Stack s;
init(&s);
printf("Enter a decimal number: ");
scanf("%d", &n);
printf("Enter the base (2-16): ");
scanf("%d", &r);
if (r < 2 || r > 16) {
printf("Invalid base\n");
return 1;
}
while (n > 0) {
int rem = n % r;
push(&s, rem);
n /= r;
}
printf("The result in base %d is: ", r);
while (!is_empty(&s)) {
int digit = pop(&s);
if (digit < 10) {
printf("%d", digit);
} else {
printf("%c", 'A' + digit - 10);
}
}
printf("\n");
return 0;
}
```
程序中使用了栈来存储转换后的数字,其中 `init` 函数用于初始化栈,`is_empty` 和 `is_full` 函数分别用于判断栈是否为空和是否已满,`push` 函数用于将元素入栈,`pop` 函数用于将元素出栈。程序首先读入一个十进制整数和要转换的进制数,然后使用循环将十进制整数转换为对应的 R 进制数,并将每一位数字压入栈中。最后从栈中依次弹出每一位数字,并输出转换后的 R 进制数。注意,在转换后的数字大于等于 10 时,需要将其转换为对应的字符。
阅读全文
相关推荐
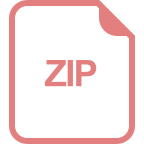















