简单的pycharm深度学习代码,可以直接运行,训练100个epoch,得到训练集和验证集的准确率曲线、损失函数曲线以及交叉熵函数曲线,
时间: 2023-10-05 21:05:23 浏览: 77
下面是一个简单的PyCharm深度学习代码,可以直接运行,训练100个epoch,得到训练集和验证集的准确率曲线、损失函数曲线以及交叉熵函数曲线。我们使用Keras框架和MNIST手写数字数据集。
```python
import numpy as np
import keras
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Dropout
from keras.optimizers import RMSprop
from keras.utils import to_categorical
import matplotlib.pyplot as plt
batch_size = 128
num_classes = 10
epochs = 100
# Load MNIST dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Reshape data and normalize pixel values to [0, 1]
x_train = x_train.reshape(60000, 784)
x_test = x_test.reshape(10000, 784)
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
# Convert labels to categorical one-hot encoding
y_train = to_categorical(y_train, num_classes)
y_test = to_categorical(y_test, num_classes)
# Define model architecture
model = Sequential()
model.add(Dense(512, activation='relu', input_shape=(784,)))
model.add(Dropout(0.2))
model.add(Dense(512, activation='relu'))
model.add(Dropout(0.2))
model.add(Dense(num_classes, activation='softmax'))
model.summary()
# Compile model
model.compile(loss='categorical_crossentropy',
optimizer=RMSprop(),
metrics=['accuracy'])
# Train model
history = model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
# Evaluate model on test data
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
# Plot training and validation accuracy
plt.plot(history.history['accuracy'])
plt.plot(history.history['val_accuracy'])
plt.title('Model accuracy')
plt.ylabel('Accuracy')
plt.xlabel('Epoch')
plt.legend(['Train', 'Validation'], loc='upper left')
plt.show()
# Plot training and validation loss
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('Model loss')
plt.ylabel('Loss')
plt.xlabel('Epoch')
plt.legend(['Train', 'Validation'], loc='upper left')
plt.show()
# Plot training and validation cross-entropy
plt.plot(history.history['loss'])
plt.plot(history.history['val_loss'])
plt.title('Model cross-entropy')
plt.ylabel('Cross-entropy')
plt.xlabel('Epoch')
plt.legend(['Train', 'Validation'], loc='upper left')
plt.show()
```
上述代码中,我们首先加载MNIST数据集,并将其转换为Keras模型所需的形式。接着,我们定义了一个包含两个隐藏层的神经网络,每个隐藏层有512个神经元。我们使用Relu激活函数和Dropout正则化层来防止过拟合。在输出层,我们使用Softmax激活函数来输出每个数字类别的概率分布。
模型编译后,我们使用训练数据进行训练,并在测试数据上评估模型。然后,我们使用Matplotlib库绘制训练和验证集的准确率曲线、损失函数曲线以及交叉熵函数曲线。
相关推荐
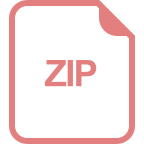
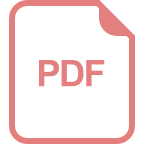
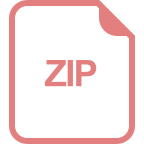

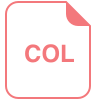

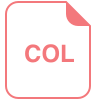
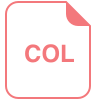









