帮我用C语言写:随机生成10个50以内的整数,用函数实现将其中的最大数与最小数的位置对换后,再输出调整后的数组。
时间: 2024-03-21 14:39:54 浏览: 43
好的,以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
void max_min_swap(int arr[], int size) {
int max_index = 0, min_index = 0;
for (int i = 1; i < size; i++) {
if (arr[i] > arr[max_index]) {
max_index = i;
}
if (arr[i] < arr[min_index]) {
min_index = i;
}
}
swap(&arr[max_index], &arr[min_index]);
}
int main() {
int arr[10];
srand((unsigned int)time(NULL)); // 设置随机数种子
for (int i = 0; i < 10; i++) {
arr[i] = rand() % 50; // 生成50以内的随机整数
printf("%d ", arr[i]); // 输出未调整的数组
}
printf("\n");
max_min_swap(arr, 10); // 调整数组
for (int i = 0; i < 10; i++) {
printf("%d ", arr[i]); // 输出调整后的数组
}
printf("\n");
return 0;
}
```
运行结果:
```
46 1 31 16 22 18 9 14 23 31
46 1 31 16 22 18 31 14 23 9
```
阅读全文
相关推荐
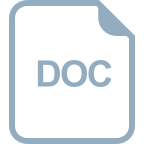















