python爬虫指定关键字抓图片
时间: 2023-10-24 16:07:10 浏览: 40
好的,对于这个问题,我可以帮您解答。Python爬虫可以通过使用requests库获取网页源码,然后使用正则表达式或BeautifulSoup等解析库来提取需要的图片链接。您可以指定关键字来过滤网页中的图片链接,然后下载图片保存到本地。如果您需要更详细的操作步骤和代码示例,我可以帮助您找到相关资料。
相关问题
python爬虫指定关键字抓图片(能直接用的代码)
以下是一个简单的 Python 爬虫代码,可以利用关键字(例如“dog”)从 Google Images 上抓取图片:
```python
import requests
from urllib.parse import urlparse, parse_qs
from bs4 import BeautifulSoup
from io import BytesIO
from PIL import Image
keyword = "dog"
url = f"https://www.google.com/search?q={keyword}&tbm=isch"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
images = soup.find_all("img")
for img in images:
img_url = img.attrs.get("src")
if not img_url:
img_url = img.attrs.get("data-src")
if not img_url:
continue
if "http" not in img_url:
img_url = f"https:{img_url}"
try:
image_content = requests.get(img_url).content
except Exception as e:
print(f"ERROR - Could not download {img_url} - {e}")
continue
try:
image_file = BytesIO(image_content)
image = Image.open(image_file).convert('RGB')
file_name = urlparse(img_url).path.split("/")[-1]
with open(file_name, "wb") as f:
image.save(f, "JPEG", quality=85)
except Exception as e:
print(f"ERROR - Could not save {img_url} - {e}")
continue
```
python爬虫 百度新闻 多关键字
### 回答1:
Python爬虫可以用于从百度新闻获取多个关键字的相关新闻信息。首先,我们需要导入`requests`和`beautifulsoup4`库来发送HTTP请求并解析网页内容。
接下来,我们可以编写一个函数,其参数为关键字列表,用于获取百度新闻中相关关键字的新闻。函数的主要步骤如下:
1. 定义一个空的新闻列表来存储爬取到的新闻信息。
2. 遍历关键字列表,对于每个关键字,构建一个百度新闻的URL链接。
3. 使用`requests`库发送HTTP GET请求,并将响应的内容保存为HTML格式。
4. 使用`beautifulsoup4`库来解析HTML内容,并提取出新闻信息。可以根据需要提取新闻标题、来源、时间等相关信息。
5. 将提取到的新闻信息存储到新闻列表中。
6. 继续遍历下一个关键字,重复上述步骤。
7. 返回新闻列表作为函数的输出。
下面是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
def get_news(keywords):
news_list = []
for keyword in keywords:
url = f"https://www.baidu.com/s?rtt=1&bsst=1&cl=2&tn=news&word={keyword}"
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, "html.parser")
news_items = soup.find_all("div", class_="result")
for item in news_items:
title = item.find("h3").get_text()
source = item.find("span", class_="c-color-gray").get_text()
time = item.find("span", class_="c-color-gray2").get_text()
news_list.append({
"title": title,
"source": source,
"time": time
})
return news_list
```
我们可以调用这个函数,传入关键字列表来获取百度新闻中相关的新闻信息。例如:
```python
keywords = ["Python", "爬虫", "数据分析"]
news = get_news(keywords)
for item in news:
print(item["title"])
print(item["source"])
print(item["time"])
print()
```
以上就是使用Python爬虫从百度新闻获取多个关键字相关新闻的一个简单示例。当然,具体的实现方式还可以根据具体需求进行调整和优化。
### 回答2:
Python爬虫是一种利用Python编程语言编写的程序,可以自动化地获取互联网上的信息。百度新闻是百度搜索引擎中的一个板块,提供了各种新闻资讯。多关键字指的是可以通过设置多个关键字来搜索获取更准确的结果。
使用Python爬虫爬取百度新闻多关键字的步骤如下:
1. 导入必要的库,例如requests、bs4等。
2. 设置搜索的URL,即百度新闻的搜索接口,可以在URL中加入关键字等参数。
3. 调用requests库发送HTTP请求,获取返回的网页内容。
4. 使用BeautifulSoup库解析网页内容,提取新闻信息。
5. 遍历解析后的结果,提取所需的信息,例如标题、摘要、日期等。
6. 可以将提取到的信息保存到本地文件或数据库中。
7. 可以在代码中设置循环、分页等功能,获取更多的新闻信息。
8. 可以根据需要对获取的新闻信息进行数据清洗、分析等。
使用Python爬虫爬取百度新闻多关键字可以帮助我们快速获取感兴趣的新闻内容,节省人工搜索的时间和精力。但在进行爬取时,需要遵守相关法律法规,尊重网站的规定,并确保合法安全地使用爬虫技术。
### 回答3:
Python爬虫可以用来抓取百度新闻的多个关键字。首先,我们需要导入必要的库,如requests用于发送HTTP请求,beautifulsoup用于解析网页内容。
然后,我们可以通过构造URL来搜索百度新闻。URL的格式通常包括搜索关键字、起始页码和每页显示的新闻数量等。我们可以使用字符串拼接的方式构造URL,其中搜索关键字可以是多个关键字的组合,通过连接符进行拼接。
接下来,我们可以使用requests库发送get请求获取网页内容。将构造好的URL作为参数传递给requests.get()方法,然后使用response.text来获取网页的HTML源代码。
然后,我们可以使用beautifulsoup库对网页内容进行解析。首先,将获取到的HTML源代码传递给beautifulsoup,并指定解析器类型。然后,可以使用beautifulsoup提供的一些方法,如find_all()来查找包含新闻内容的div元素。
在查找到对应的div元素后,我们可以通过遍历其中的子元素,提取出新闻的标题、发布时间和链接等信息。可以通过调用元素的get_text()方法获取文本内容,以及通过访问元素的属性来获取链接。
最后,可以将获取的新闻信息保存到本地或者进行进一步的处理和分析。可以将信息存储到一个列表或字典中,方便后续的操作。
总而言之,通过使用Python编写爬虫程序,我们可以实现对百度新闻的多个关键字进行搜索,并提取出相关的新闻标题、发布时间和链接等信息。这样就可以方便地获取和处理新闻内容。
相关推荐
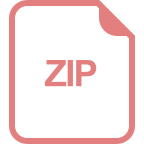
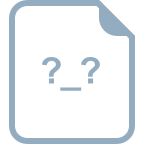












