用Java写超市购物程序设计
时间: 2024-11-03 16:18:34 浏览: 31
在Java中编写一个超市购物程序设计,可以包括以下几个步骤:
1. **商品类(Product)**:作为基础模型,包含属性如名称、价格和库存等。例如:
```java
public class Product {
private String name;
private double price;
private int stock;
// 构造函数、getter和setter方法
public Product(String name, double price, int stock) {
this.name = name;
this.price = price;
this.stock = stock;
}
}
```
2. **购物车类(ShoppingCart)**:用于存储商品并实现添加、删除和计算总价的功能。
```java
import java.util.ArrayList;
import java.util.List;
public class ShoppingCart {
private List<Product> items;
public ShoppingCart() {
items = new ArrayList<>();
}
// 添加商品、删除商品、计算总金额的方法
public void addItem(Product product) {
items.add(product);
}
public void removeItem(Product product) {
items.remove(product);
}
public double calculateTotal() {
double total = 0;
for (Product item : items) {
total += item.getPrice();
}
return total;
}
}
```
3. **顾客类(Customer)**:可以有姓名和购物车属性。
```java
public class Customer {
private String name;
private ShoppingCart cart;
public Customer(String name) {
this.name = name;
this.cart = new ShoppingCart();
}
// 添加购物车商品的方法
public void addToCart(Product product) {
cart.addItem(product);
}
}
```
4. **主程序入口(Main Class)**:测试上述类的功能。
```java
public class Main {
public static void main(String[] args) {
Customer customer = new Customer("Alice");
Product apple = new Product("苹果", 5.0, 10);
customer.addToCart(apple);
System.out.println("顾客 " + customer.getName() + " 的购物车总额:" + customer.cart.calculateTotal());
}
}
```
阅读全文
相关推荐
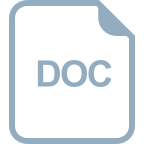
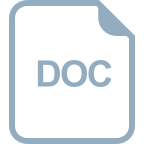
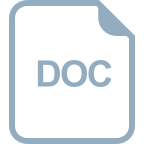
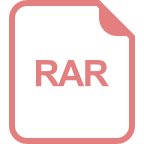
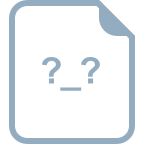


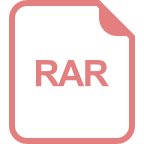
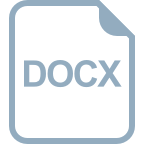
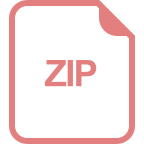
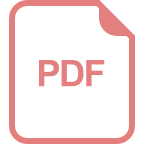
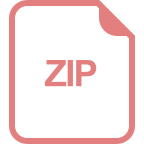
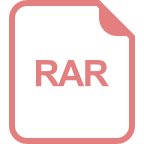
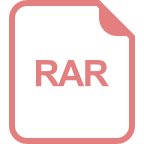
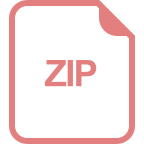
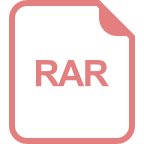
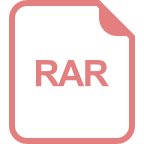
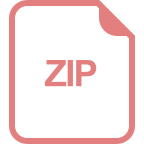
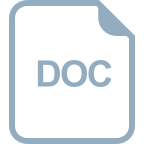