实现一个算法来识别一个字符串是否是另一个字符串的排列第一行为字符串A第二行为字符串B字符串长度不超过100
时间: 2024-10-11 19:04:28 浏览: 24
要实现一个算法来判断两个字符串A和B是否为彼此的排列(即字符相同但顺序不同),你可以使用哈希表(如字典或关联数组)来存储每个字符串中的字符及其出现次数。然后,比较两个哈希表是否相等。这里是一个简单的C语言示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
// 函数用于计算字符出现次数并更新哈希表
void count_chars(char* str, int* char_count) {
for (int i = 0; str[i] != '\0'; i++) {
(*char_count)[str[i]]++;
}
}
// 检查两个哈希表是否相等
bool is_permutation(char* a, char* b) {
int a_char_count[256] = {0}; // 假设ASCII字符集大小
int b_char_count[256] = {0};
count_chars(a, a_char_count);
count_chars(b, b_char_count);
for (int i = 0; i < 256; i++) {
if (a_char_count[i] != b_char_count[i]) {
return false;
}
}
return true;
}
int main() {
char stringA[101], stringB[101];
printf("Enter string A: ");
fgets(stringA, sizeof(stringA), stdin); // 注意去掉末尾的换行符
stringA[strcspn(stringA, "\n")] = '\0';
printf("Enter string B: ");
fgets(stringB, sizeof(stringB), stdin);
stringB[strcspn(stringB, "\n")] = '\0';
bool are_permutations = is_permutation(stringA, stringB);
if (are_permutations) {
printf("Strings A and B are permutations of each other.\n");
} else {
printf("Strings A and B are not permutations of each other.\n");
}
return 0;
}
```
在这个程序中,`count_chars`函数计算字符串中的字符频率,而`is_permutation`函数检查这两个哈希表是否相等。如果相等,则说明字符串A和B是排列。
阅读全文
相关推荐
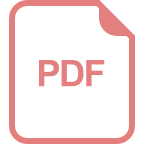
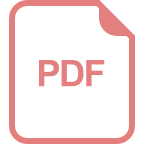
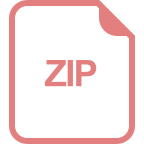















