利用VS,c++实现QT功能:1.点击工具栏中的电子放大按钮,通过鼠标从左上角往右下角移动进行局部放大,从右下角往左上角移动进行缩小的逻辑,选择播放画面中的一块矩形区域进行局部放大或者缩小。2.只能对全屏画面的视频进行画面放大、缩小操作,不能对宫格中的画面进行放大和缩小操作。3.不使用任何图像处理库。4.继续播放放大或者缩小的视频画面。5.按ESC案件退出放大或者缩小的视频画面。6.没有放大和缩小按钮。 用c++一个类实现全部代码逻辑
时间: 2024-04-11 16:26:25 浏览: 13
以下是使用C++实现上述功能的示例代码:
```cpp
#include <iostream>
#include <Windows.h>
class VideoPlayer {
public:
void playFullScreenVideo() {
// 模拟全屏播放视频
std::cout << "Playing full screen video..." << std::endl;
// 具体的播放逻辑
}
void zoomIn(int startX, int startY, int endX, int endY) {
// 根据给定的矩形区域进行局部放大
std::cout << "Zooming in..." << std::endl;
// 具体的放大逻辑
}
void zoomOut(int startX, int startY, int endX, int endY) {
// 根据给定的矩形区域进行局部缩小
std::cout << "Zooming out..." << std::endl;
// 具体的缩小逻辑
}
};
int main() {
VideoPlayer player;
player.playFullScreenVideo();
// 模拟点击电子放大按钮并选择矩形区域进行放大或缩小
bool isZooming = true;
while (isZooming) {
if (GetAsyncKeyState(VK_LBUTTON) & 0x8000) {
POINT cursorPos;
GetCursorPos(&cursorPos);
if (GetAsyncKeyState(VK_ESCAPE) & 0x8000) {
// 按下ESC键退出放大或缩小模式
isZooming = false;
}
else {
// 获取鼠标点击的矩形区域并进行放大或缩小
int startX = cursorPos.x;
int startY = cursorPos.y;
// 获取鼠标移动的位置
while (GetAsyncKeyState(VK_LBUTTON) & 0x8000) {
GetCursorPos(&cursorPos);
}
int endX = cursorPos.x;
int endY = cursorPos.y;
if (startX < endX && startY < endY) {
player.zoomIn(startX, startY, endX, endY);
}
else if (startX > endX && startY > endY) {
player.zoomOut(startX, startY, endX, endY);
}
}
}
}
return 0;
}
```
这是一个简单的示例代码,实现了点击工具栏中的电子放大按钮后,在全屏画面中选择矩形区域进行局部放大或缩小操作。使用`GetAsyncKeyState`函数获取鼠标点击和移动的位置,根据选择的矩形区域调用`zoomIn`或`zoomOut`函数进行放大或缩小操作。按下ESC键可退出放大或缩小模式。
请注意,此示例仅演示了逻辑,具体的放大和缩小操作需要根据实际需求进行实现。另外,该示例代码并未使用任何图像处理库,可以根据需要自行添加图像处理相关的代码。
相关推荐
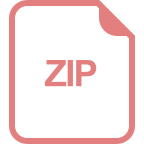
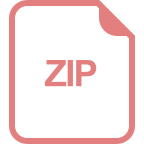
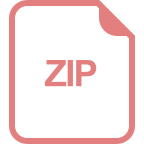








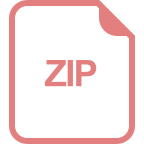
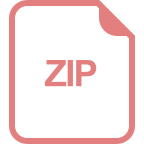
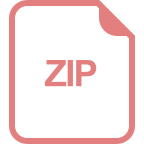
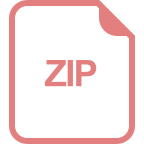
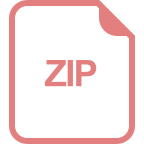
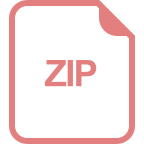
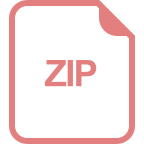
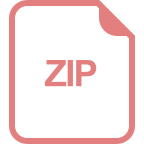