ffmpeg改变分辨率 帧高比
时间: 2024-12-28 09:10:51 浏览: 0
FFmpeg是一个强大的跨平台多媒体处理工具,它可以用来转换视频的分辨率和帧率,也就是改变视频的画面尺寸和每秒显示的帧数。如果你想要通过FFmpeg将视频从一种分辨率调整到另一种,并保持原始的宽高比(Aspect Ratio),可以使用如下的命令格式:
```sh
ffmpeg -i input.mp4 -vf "scale=-1:height_in_desired" -c:a copy output.mp4
```
在这个例子中:
- `-i input.mp4` 指定了输入文件。
- `-vf "scale=-1:height_in_desired"` 使用了Video Filter(滤镜)中的 `scale` ,其中 `-1` 表示宽度会自动计算以保持原始宽高比,`height_in_desired` 是你想达到的新高度。
- `-c:a copy` 保留原音频质量,不进行编码,如果需要重新编码音频,这里可以替换为其他选项。
- `output.mp4` 是输出的目标文件。
如果你想改变帧率(Frame Rate),则可以添加 `-r frames_per_second` 参数:
```sh
ffmpeg -vf ... -c:a copy output.mp4
```
在这里,`new_frame_rate` 是目标帧率。
相关问题
Ffmpeg怎么通过在编解码过程中手动改变共识内存中的参数实时改变分辨率
可以通过在编解码过程中使用FFmpeg的API来手动更改共识内存中的参数,从而实时改变视频分辨率。具体步骤如下:
1. 打开输入文件并获取视频流信息。
```c++
AVFormatContext *inputContext = NULL;
int ret = avformat_open_input(&inputContext, inputUrl, NULL, NULL);
if (ret < 0) {
// 打开输入文件失败
return;
}
ret = avformat_find_stream_info(inputContext, NULL);
if (ret < 0) {
// 获取视频流信息失败
return;
}
int videoStreamIndex = -1;
for (int i = 0; i < inputContext->nb_streams; i++) {
AVStream *stream = inputContext->streams[i];
if (stream->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
// 没有找到视频流
return;
}
AVCodecParameters *codecpar = inputContext->streams[videoStreamIndex]->codecpar;
```
2. 打开输出文件并设置视频编码器。
```c++
AVFormatContext *outputContext = NULL;
ret = avformat_alloc_output_context2(&outputContext, NULL, NULL, outputUrl);
if (ret < 0) {
// 打开输出文件失败
return;
}
AVCodec *videoCodec = avcodec_find_encoder(codecpar->codec_id);
if (videoCodec == NULL) {
// 找不到视频编码器
return;
}
AVCodecContext *codecContext = avcodec_alloc_context3(videoCodec);
if (codecContext == NULL) {
// 分配编码器上下文失败
return;
}
codecContext->codec_id = codecpar->codec_id;
codecContext->bit_rate = codecpar->bit_rate;
codecContext->width = codecpar->width; // 设置初始分辨率
codecContext->height = codecpar->height;
codecContext->time_base = codecpar->time_base;
codecContext->framerate = codecpar->framerate;
codecContext->pix_fmt = videoCodec->pix_fmts[0];
avcodec_parameters_to_context(codecContext, codecpar);
```
3. 打开视频编码器并写入文件头。
```c++
ret = avcodec_open2(codecContext, videoCodec, NULL);
if (ret < 0) {
// 打开编码器失败
return;
}
AVStream *videoStream = avformat_new_stream(outputContext, videoCodec);
if (videoStream == NULL) {
// 创建视频流失败
return;
}
avcodec_parameters_from_context(videoStream->codecpar, codecContext);
av_dump_format(outputContext, 0, outputUrl, 1);
ret = avio_open(&outputContext->pb, outputUrl, AVIO_FLAG_WRITE);
if (ret < 0) {
// 打开输出文件失败
return;
}
ret = avformat_write_header(outputContext, NULL);
if (ret < 0) {
// 写入文件头失败
return;
}
```
4. 循环读取视频帧并编码。
```c++
AVPacket *packet = av_packet_alloc();
AVFrame *frame = av_frame_alloc();
int64_t pts = 0;
while (av_read_frame(inputContext, packet) >= 0) {
if (packet->stream_index == videoStreamIndex) {
ret = avcodec_send_packet(codecContext, packet);
if (ret < 0) {
// 发送数据到编码器失败
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecContext, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
// 编码器需要更多数据或已经结束
break;
}
if (ret < 0) {
// 接收编码器输出失败
break;
}
// 修改分辨率
codecContext->width = newWidth;
codecContext->height = newHeight;
frame->width = newWidth;
frame->height = newHeight;
// 编码视频帧
frame->pts = pts;
pts += av_rescale_q(frame->pkt_dts, inputContext->streams[videoStreamIndex]->time_base, codecContext->time_base);
ret = avcodec_send_frame(codecContext, frame);
if (ret < 0) {
// 发送数据到编码器失败
break;
}
while (ret >= 0) {
ret = avcodec_receive_packet(codecContext, packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
// 编码器需要更多数据或已经结束
break;
}
if (ret < 0) {
// 接收编码器输出失败
break;
}
// 写入视频帧
av_packet_rescale_ts(packet, codecContext->time_base, videoStream->time_base);
av_interleaved_write_frame(outputContext, packet);
av_packet_unref(packet);
}
}
}
av_packet_unref(packet);
}
```
5. 写入文件尾并释放资源。
```c++
av_write_trailer(outputContext);
avcodec_free_context(&codecContext);
avformat_close_input(&inputContext);
avformat_free_context(outputContext);
av_frame_free(&frame);
av_packet_free(&packet);
```
如何使用FFmpeg实现视频和音频的转码,并确保输出媒体的帧率和分辨率符合特定要求?
在多媒体处理领域,FFmpeg是一个功能全面的工具,它能够帮助你完成从简单到复杂的视频和音频转码任务。对于你提出的问题,要实现视频和音频的转码并调整帧率和分辨率,首先需要了解基本的FFmpeg命令行结构和一些关键参数的作用。
参考资源链接:[FFmpeg教程:视频音频转码与解码解析](https://wenku.csdn.net/doc/68z3kqvhan?spm=1055.2569.3001.10343)
使用FFmpeg进行视频转码的基本命令格式如下:
```bash
ffmpeg -i input.mp4 -vf scale=1920:1080 -r 30 output.mp4
```
在这个命令中:
- `-i input.mp4` 指定了输入文件。
- `-vf scale=1920:1080` 是一个视频过滤器(video filter),用于将视频的分辨率调整为1920x1080,其中`scale`是过滤器的名称,`1920:1080`是目标分辨率。
- `-r 30` 设置输出视频的帧率为每秒30帧。
- `output.mp4` 指定了输出文件的名称。
对于音频转码,可以使用类似的方法添加音频相关参数:
```bash
ffmpeg -i input.mp4 -vf scale=1920:1080 -r 30 -acodec aac -ar 44100 output.mp4
```
在这里 `-acodec aac` 指定了使用AAC编码器进行音频编码,`-ar 44100` 设置音频的采样率为44100Hz。
要确保视频和音频同步,FFmpeg会根据输入流的时间戳自动处理。如果需要调整同步,可以通过`-itsoffset`参数为音频或视频流设置时间偏移量,或者在转码后使用`ffmpeg`的`-filter_complex`选项来手动同步音视频流。
掌握这些基本的转码命令之后,你可以根据实际需求进行调整,例如改变视频和音频的编码格式、比特率等。为了深入了解FFmpeg的更多高级用法,建议参考这本《FFmpeg教程:视频音频转码与解码解析》,它将为你提供更全面的知识和实践案例,帮助你在多媒体处理的道路上不断进步。
参考资源链接:[FFmpeg教程:视频音频转码与解码解析](https://wenku.csdn.net/doc/68z3kqvhan?spm=1055.2569.3001.10343)
阅读全文
相关推荐
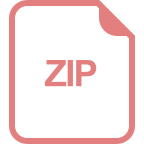
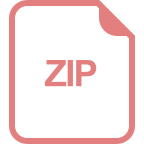
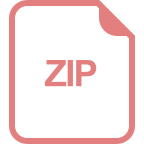
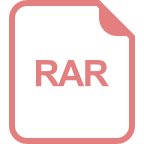
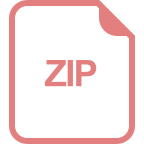
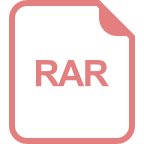
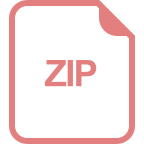
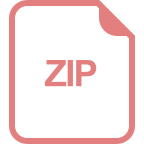
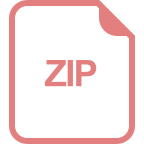
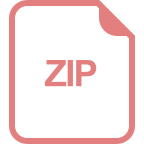
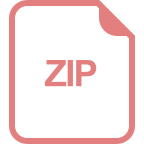
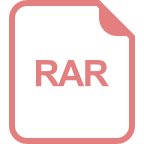
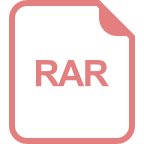
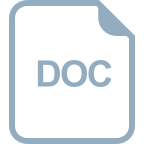
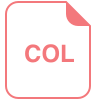
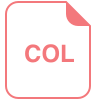
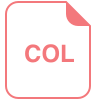