qstring使用正则
时间: 2024-02-21 08:53:01 浏览: 68
QString使用正则表达式提供了多个接口来进行匹配和操作。其中,QRegularExpression是Qt 5.0引入的新类,修复了许多之前版本中QRegExp存在的问题,并且在功能上与QRegExp兼容。因此,推荐使用QRegularExpression。
你可以使用QRegularExpression的contains方法来判断一个字符串中是否存在与正则表达式匹配的部分。例如,你可以创建一个QRegularExpression对象,然后调用contains方法传入待匹配的字符串来进行判断。
另外,你也可以使用QRegularExpression对字符串进行替换、提取等操作。QRegularExpression提供了replace和match方法,分别用于替换和提取字符串中与正则表达式匹配的部分。
相关问题
QString 使用正则表达式
以下是使用正则表达式的QString的例子:
1. 使用QString的contains()方法来检查字符串是否匹配正则表达式:
```cpp
QString str = "Hello, World!";
QString pattern = "[A-Za-z]+";
QRegExp rx(pattern);
if (str.contains(rx)) {
qDebug() << "String matches the pattern";
} else {
qDebug() << "String does not match the pattern";
}
```
2. 使用QString的replace()方法来替换匹配正则表达式的部分:
```cpp
QString str = "Hello, World!";
QString pattern = "[A-Za-z]+";
QRegExp rx(pattern);
str.replace(rx, "Replacement");
qDebug() << str; // 输出:Replacement, Replacement!
```
3. 使用QString的indexIn()方法来查找第一个匹配正则表达式的位置:
```cpp
QString str = "Hello, World!";
QString pattern = "[A-Za-z]+";
QRegExp rx(pattern);
int index = rx.indexIn(str);
if (index != -1) {
qDebug() << "Pattern found at index" << index;
} else {
qDebug() << "Pattern not found";
}
```
QString 的正则表达式用法
QString 类提供了一系列正则表达式的方法,可以用来匹配、替换、捕获等操作。以下是一些常见的用法:
1. 字符串匹配
```cpp
QString str = "hello world";
QRegExp regex("world");
if (regex.indexIn(str) != -1) {
qDebug() << "Matched";
} else {
qDebug() << "Not matched";
}
```
2. 捕获子串
```cpp
QString str = "hello world";
QRegExp regex("(.*) world");
if (regex.indexIn(str) != -1) {
qDebug() << "Matched";
qDebug() << regex.cap(1); // 输出 "hello"
} else {
qDebug() << "Not matched";
}
```
3. 替换字符串
```cpp
QString str = "hello world";
QRegExp regex("world");
str.replace(regex, "Qt");
qDebug() << str; // 输出 "hello Qt"
```
4. 判断字符串是否符合规则
```cpp
QString str = "password123";
QRegExp regex("^[a-zA-Z]\\w{5,17}$"); // 匹配以字母开头,长度为6-18的字符串
if (regex.exactMatch(str)) {
qDebug() << "Valid password";
} else {
qDebug() << "Invalid password";
}
```
5. 分割字符串
```cpp
QString str = "hello,world,Qt";
QStringList list = str.split(QRegExp("[,]"));
qDebug() << list; // 输出 ["hello", "world", "Qt"]
```
以上只是一些常见用法,Qt 的正则表达式支持更多的功能,可以参考文档进行学习和使用。
阅读全文
相关推荐
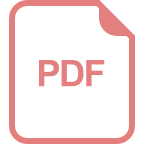
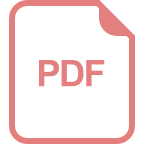
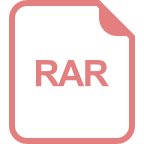













