请在两个变量中举个例子
时间: 2024-03-21 15:38:37 浏览: 43
好的,这里提供一个例子:
```c
#include <stdio.h>
int main() {
int a, b;
printf("请输入两个整数:\n");
scanf("%d%d", &a, &b);
printf("您输入的两个整数分别是:%d和%d\n", a, b);
return 0;
}
```
上面这段代码中,使用了 scanf 函数来从标准输入流中读取用户输入的两个整数。其中,&a 和 &b 分别表示 a 和 b 的地址,可以通过这种方式将用户输入的值赋给 a 和 b。
而如果使用 fscanf_s 函数的话,可以将上面的代码改写为:
```c
#include <stdio.h>
int main() {
int a, b;
FILE* fp;
fopen_s(&fp, "input.txt", "r");
fscanf_s(fp, "%d%d", &a, &b);
printf("您输入的两个整数分别是:%d和%d\n", a, b);
fclose(fp);
return 0;
}
```
这里使用了 fscanf_s 函数从文件中读取两个整数,其中 fp 是文件指针,通过 fopen_s 函数打开文件。需要注意的是,fscanf_s 函数的第一个参数是文件指针,而不是标准输入流(stdin)。
相关问题
在操作系统中举一个关于临界区的例子,用代码实现
在操作系统中,临界区通常用于保护共享资源,防止多个线程同时访问导致数据不一致。一个经典的例子是银行账户余额,如果多个进程试图读取和修改这个余额,就需要确保在同一时间只有一个进程可以操作。
例如,在单处理器系统下,我们可以使用一种简单的互斥量(Mutex)机制来创建一个临界区:
```c
#include <pthread.h>
#include <stdio.h>
// 共享变量
int balance = 0;
// 临界区函数
void critical_section(int amount) {
pthread_mutex_lock(&mutex); // 获取锁
try {
printf("Process %d is modifying the balance: %d\n", pthread_self(), balance);
// 操作平衡
balance += amount;
if (balance < 0) {
printf("Balance cannot be negative: %d\n", balance);
} else {
printf("New balance: %d\n", balance);
}
} catch (...) {
printf("An error occurred while modifying the balance.\n");
}
pthread_mutex_unlock(&mutex); // 释放锁
}
int main() {
pthread_t thread1, thread2; // 创建两个线程
pthread_mutex_init(&mutex, NULL); // 初始化互斥量
// 启动线程并分别处理临界区
pthread_create(&thread1, NULL, &critical_section, (void*)10);
pthread_create(&thread2, NULL, &critical_section, (void*)5);
// 等待所有线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&mutex); // 销毁互斥量
return 0;
}
```
在这个例子中,`critical_section` 函数被视为临界区,其中对 `balance` 的操作只能由持有互斥量(通过 `pthread_mutex_lock()` 和 `pthread_mutex_unlock()`)的线程执行。这样就保证了任何时候只有一个线程能更新余额,避免了并发问题。
阅读全文
相关推荐
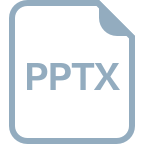
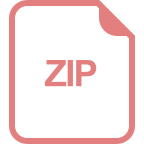
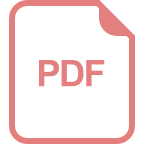
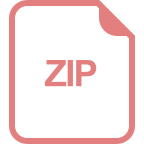
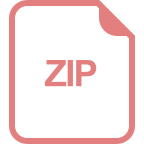
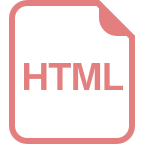
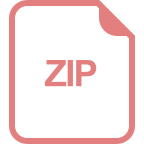
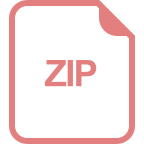
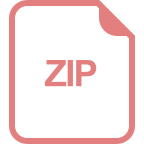
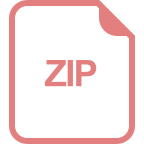
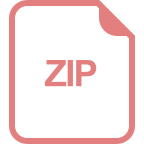
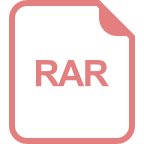