opus sample
时间: 2023-10-27 17:08:03 浏览: 58
Opus 是一种开源音频编解码器,适用于音频流传输和储存。以下是 Opus 编码和解码的示例代码:
编码:
```c
#include <opus/opus.h>
int main() {
int error;
OpusEncoder *encoder;
int32_t sample_rate = 48000;
int channels = 2;
int application = OPUS_APPLICATION_AUDIO;
int max_payload_bytes = 4000;
encoder = opus_encoder_create(sample_rate, channels, application, &error);
if (error != OPUS_OK) {
fprintf(stderr, "failed to create encoder: %s\n", opus_strerror(error));
return -1;
}
error = opus_encoder_ctl(encoder, OPUS_SET_MAX_PAYLOAD_BYTES(max_payload_bytes));
if (error != OPUS_OK) {
fprintf(stderr, "failed to set max payload bytes: %s\n", opus_strerror(error));
return -1;
}
int16_t pcm_frame[960 * channels];
uint8_t compressed_frame[max_payload_bytes];
int compressed_size;
/* read a frame of audio data from somewhere */
while (1) {
/* encode the frame */
compressed_size = opus_encode(encoder, pcm_frame, 960, compressed_frame, max_payload_bytes);
if (compressed_size < 0) {
fprintf(stderr, "opus_encode failed: %s\n", opus_strerror(compressed_size));
return -1;
}
/* send the compressed data over the network */
send_data_to_network(compressed_frame, compressed_size);
}
opus_encoder_destroy(encoder);
}
```
解码:
```c
#include <opus/opus.h>
int main() {
int error;
OpusDecoder *decoder;
int32_t sample_rate = 48000;
int channels = 2;
int max_payload_bytes = 4000;
decoder = opus_decoder_create(sample_rate, channels, &error);
if (error != OPUS_OK) {
fprintf(stderr, "failed to create decoder: %s\n", opus_strerror(error));
return -1;
}
error = opus_decoder_ctl(decoder, OPUS_SET_MAX_PAYLOAD_BYTES(max_payload_bytes));
if (error != OPUS_OK) {
fprintf(stderr, "failed to set max payload bytes: %s\n", opus_strerror(error));
return -1;
}
uint8_t compressed_frame[max_payload_bytes];
int compressed_size;
int16_t pcm_frame[960 * channels];
int pcm_size;
/* receive a compressed frame of audio data from the network */
while (1) {
/* decode the frame */
pcm_size = opus_decode(decoder, compressed_frame, compressed_size, pcm_frame, 960, 0);
if (pcm_size < 0) {
fprintf(stderr, "opus_decode failed: %s\n", opus_strerror(pcm_size));
return -1;
}
/* play the decoded audio */
play_audio(pcm_frame, pcm_size);
}
opus_decoder_destroy(decoder);
}
```
相关推荐
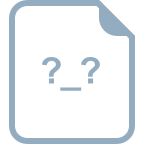
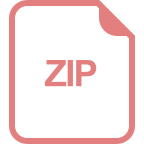
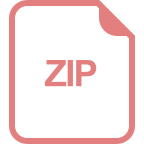














