如何在Java中使用监听事件
时间: 2023-07-09 15:46:13 浏览: 85
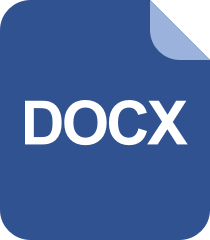
【JavaScript源代码】JavaScript给事件委托批量添加事件监听详细流程.docx
在 Java 中使用监听事件的方法有很多,这里介绍其中两种常用的方法:
1. 使用内部类实现监听器
使用内部类来实现监听器是 Java 中最常见的方法之一。在这种方法中,我们可以在事件源类中定义一个内部类,该内部类实现事件监听器接口,然后将其实例注册到事件源中。当事件发生时,事件源会调用该内部类的方法来响应事件。
举个例子,假设我们有一个按钮类,我们要在按钮上添加一个监听器,当用户单击按钮时,会触发事件。我们可以使用下面的代码来实现:
```java
import java.awt.*;
import java.awt.event.*;
public class ButtonExample {
private Frame mainFrame;
private Button btn;
public ButtonExample(){
prepareGUI();
}
public static void main(String[] args){
ButtonExample be = new ButtonExample();
be.showEventDemo();
}
private void prepareGUI(){
mainFrame = new Frame("Java Button Example");
mainFrame.setSize(300,150);
mainFrame.setLayout(new FlowLayout());
mainFrame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent windowEvent){
System.exit(0);
}
});
btn = new Button("Click me!");
mainFrame.add(btn);
mainFrame.setVisible(true);
}
private void showEventDemo(){
// 使用内部类来实现 ActionListener 接口
class ButtonClickListener implements ActionListener{
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked!");
}
}
btn.addActionListener(new ButtonClickListener());
}
}
```
在这个示例中,我们定义了一个内部类 ButtonClickListener,该类实现了 ActionListener 接口。在 showEventDemo() 方法中,我们将一个 ButtonClickListener 类型的实例注册到按钮中,当用户单击按钮时,ButtonClickListener 类的 actionPerformed() 方法就会被调用。
2. 使用 Lambda 表达式实现监听器
Java 8 引入了 Lambda 表达式,使得代码变得更加简洁和易读。Lambda 表达式可以用来实现事件监听器,可以将其视为匿名类的一种简化形式。
举个例子,我们可以使用 Lambda 表达式来实现上面的示例,代码如下:
```java
import java.awt.*;
import java.awt.event.*;
public class ButtonExample {
private Frame mainFrame;
private Button btn;
public ButtonExample(){
prepareGUI();
}
public static void main(String[] args){
ButtonExample be = new ButtonExample();
be.showEventDemo();
}
private void prepareGUI(){
mainFrame = new Frame("Java Button Example");
mainFrame.setSize(300,150);
mainFrame.setLayout(new FlowLayout());
mainFrame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent windowEvent){
System.exit(0);
}
});
btn = new Button("Click me!");
mainFrame.add(btn);
mainFrame.setVisible(true);
}
private void showEventDemo(){
// 使用 Lambda 表达式来实现 ActionListener 接口
btn.addActionListener(e -> System.out.println("Button clicked!"));
}
}
```
在这个示例中,我们使用 Lambda 表达式来实现 ActionListener 接口,可以更加简洁地处理事件。
阅读全文
相关推荐
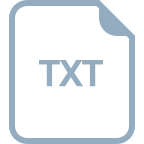
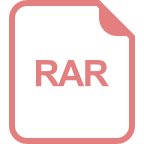
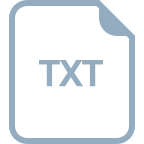
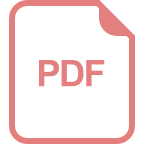
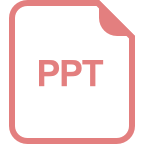
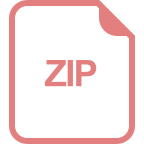
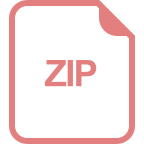
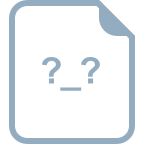
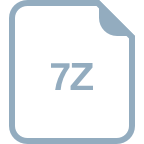
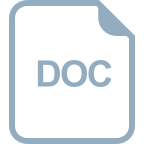
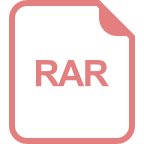
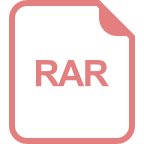