在springboot,mybatis-plus项目中,应该怎么样实现上述功能,请详细说明步骤
时间: 2023-05-11 18:05:58 浏览: 56
您好,对于在 Spring Boot 和 MyBatis-Plus 项目中实现上述功能,您可以按照以下步骤进行操作:
1. 首先,您需要在您的项目中添加 MyBatis-Plus 的依赖,可以在 pom.xml 文件中添加以下代码:
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3.1</version>
</dependency>
```
2. 然后,您需要创建一个实体类,用于映射数据库中的表,可以使用 MyBatis-Plus 提供的 @TableName 和 @TableId 注解来指定表名和主键:
```
@TableName("user")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
// 省略 getter 和 setter 方法
}
```
3. 接下来,您需要创建一个 Mapper 接口,用于定义对数据库的操作,可以使用 MyBatis-Plus 提供的 BaseMapper 接口来继承:
```
public interface UserMapper extends BaseMapper<User> {
}
```
4. 然后,您需要在 application.properties 或 application.yml 文件中配置数据库连接信息,例如:
```
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
5. 最后,您可以在您的业务代码中使用 UserMapper 接口来进行数据库操作,例如:
```
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public List<User> listUsers() {
return userMapper.selectList(null);
}
public User getUserById(Long id) {
return userMapper.selectById(id);
}
public void addUser(User user) {
userMapper.insert(user);
}
public void updateUser(User user) {
userMapper.updateById(user);
}
public void deleteUser(Long id) {
userMapper.deleteById(id);
}
}
```
以上就是在 Spring Boot 和 MyBatis-Plus 项目中实现对数据库的增删改查操作的步骤。希望能对您有所帮助。
相关推荐
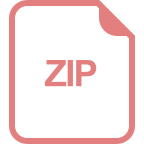
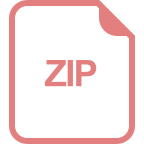
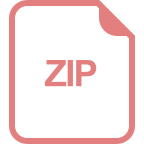














