k最近邻分类算法实现代码
时间: 2023-07-05 08:08:10 浏览: 111
下面是一个简单的k最近邻分类算法实现的代码示例:
```python
import numpy as np
class KNN:
def __init__(self, k=3):
self.k = k
def fit(self, X, y):
self.X_train = X
self.y_train = y
def predict(self, X):
y_pred = []
for x in X:
distances = np.sqrt(np.sum((self.X_train - x) ** 2, axis=1))
nearest_neighbors = np.argsort(distances)[:self.k]
nearest_labels = self.y_train[nearest_neighbors]
y_pred.append(np.bincount(nearest_labels).argmax())
return np.array(y_pred)
```
其中, `fit` 方法用于训练模型, `predict` 方法用于预测新的数据。该实现使用欧氏距离作为距离度量,并且采用简单的多数表决法进行分类。
相关问题
用C语言写出k最近邻分类算法实现代码
以下是用C语言实现k最近邻分类算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
typedef struct {
double x;
double y;
int label;
} Point;
int main() {
int k, n;
printf("Enter the value of k: ");
scanf("%d", &k);
printf("Enter the number of points: ");
scanf("%d", &n);
Point* points = (Point*) malloc(n * sizeof(Point));
printf("Enter the coordinates and label of each point:\n");
for (int i = 0; i < n; i++) {
scanf("%lf %lf %d", &points[i].x, &points[i].y, &points[i].label);
}
// Classify new points
int q;
printf("Enter the number of new points to classify: ");
scanf("%d", &q);
printf("Enter the coordinates of each new point:\n");
for (int i = 0; i < q; i++) {
double x, y;
printf("Point %d: ", i+1);
scanf("%lf %lf", &x, &y);
// Compute distance between new point and all points in dataset
double distances[n];
for (int j = 0; j < n; j++) {
double dx = x - points[j].x;
double dy = y - points[j].y;
distances[j] = sqrt(dx*dx + dy*dy);
}
// Find k nearest points
int indices[n];
for (int j = 0; j < n; j++) {
indices[j] = j;
}
for (int j = 0; j < k; j++) {
int min_index = j;
for (int l = j+1; l < n; l++) {
if (distances[l] < distances[min_index]) {
min_index = l;
}
}
double temp_dist = distances[j];
distances[j] = distances[min_index];
distances[min_index] = temp_dist;
int temp_index = indices[j];
indices[j] = indices[min_index];
indices[min_index] = temp_index;
}
// Classify new point by majority vote of k nearest points
int label_counts[3] = {0, 0, 0};
for (int j = 0; j < k; j++) {
int label = points[indices[j]].label;
label_counts[label-1]++;
}
int max_count = 0;
int max_label = 1;
for (int j = 0; j < 3; j++) {
if (label_counts[j] > max_count) {
max_count = label_counts[j];
max_label = j+1;
}
}
printf("Classified as label %d\n", max_label);
}
free(points);
return 0;
}
```
这里实现了一个简单的k最近邻分类器,可以处理二维空间中的点,并将它们分为三个类别。用户可以输入要分类的新点的坐标,并得到它所属的类别。注意,这里假设数据集中有且仅有三个类别,因此在实际应用中可能需要根据具体情况作出调整。
python 读取csv文件数据 K-最近邻分类算法算法分类
读取CSV文件可以使用Python内置的csv模块,K-最近邻分类算法可以使用scikit-learn库中的KNeighborsClassifier类实现。下面是一个简单的示例代码:
```python
import csv
import numpy as np
from sklearn.neighbors import KNeighborsClassifier
# 读取CSV文件数据
with open('data.csv', newline='') as csvfile:
rows = csv.reader(csvfile)
data = list(rows)
# 将数据转换为numpy数组
data = np.array(data).astype(float)
# 分离特征和标签
X = data[:, :-1]
y = data[:, -1]
# 创建K-最近邻分类器
knn = KNeighborsClassifier(n_neighbors=3)
# 训练分类器
knn.fit(X, y)
# 预测新样本
x_new = np.array([[1.2, 2.3, 4.5]])
prediction = knn.predict(x_new)
print(prediction)
```
在这个示例中,我们首先使用csv模块读取了一个名为data.csv的CSV文件,然后将数据转换为numpy数组。接着,我们将特征和标签分离,并使用KNeighborsClassifier类创建了一个K-最近邻分类器。最后,我们用fit方法训练分类器,并用predict方法对新样本进行预测。
阅读全文
相关推荐
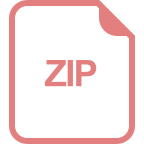
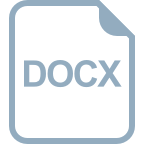
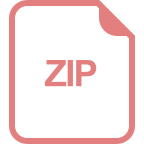
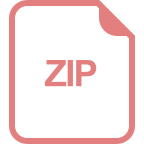
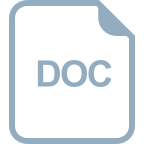
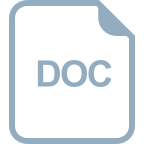
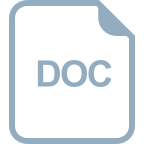
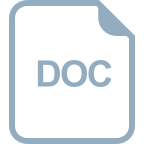
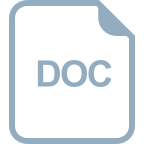
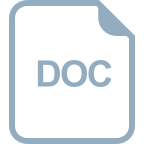
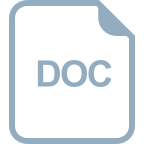
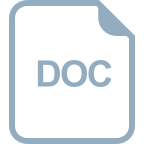
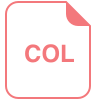