linux打印线程ID
时间: 2023-10-13 08:18:16 浏览: 65
在Linux系统中,可以使用pthread_self()函数来获取当前线程的线程ID。该函数定义在pthread.h头文件中,使用时需要链接libpthread库。
以下是一个示例程序,打印出当前线程的线程ID:
```c
#include <pthread.h>
#include <stdio.h>
void* thread_func(void* arg)
{
printf("Thread ID: %lu\n", pthread_self());
return NULL;
}
int main()
{
pthread_t tid;
pthread_create(&tid, NULL, thread_func, NULL);
pthread_join(tid, NULL);
return 0;
}
```
其中,pthread_t类型是线程ID的数据类型,pthread_create()函数用于创建一个新线程,pthread_join()函数用于等待线程结束。在线程函数thread_func中,使用pthread_self()函数打印出当前线程的线程ID。注意,%lu是用于格式化无符号长整型的printf()格式控制符。
相关问题
linux下打印执行线程的cpu
在Linux下,可以使用`pthread_getaffinity_np`函数获取当前线程执行所在的CPU。具体用法如下:
```c
#include <pthread.h>
#include <sched.h>
void print_thread_cpu()
{
cpu_set_t cpuset;
pthread_t thread = pthread_self();
int rc = pthread_getaffinity_np(thread, sizeof(cpu_set_t), &cpuset);
if (rc == 0) {
printf("Thread is running on CPU(s): ");
for (int i = 0; i < CPU_SETSIZE; i++) {
if (CPU_ISSET(i, &cpuset)) {
printf("%d ", i);
}
}
printf("\n");
}
}
```
在上面的代码中,`pthread_self()`函数用于获取当前线程的ID,然后调用`pthread_getaffinity_np`函数获取线程所在的CPU。如果`pthread_getaffinity_np`函数执行成功,就可以通过`CPU_ISSET`宏判断CPU集合中哪些CPU被分配给了当前线程,从而得到线程所在的CPU编号。
注意,`pthread_getaffinity_np`函数可能会返回`EINVAL`错误,这种情况下需要检查线程ID的有效性。另外,`CPU_ISSET`宏在`sched.h`头文件中定义。
linux 多线程求和
以下是一个简单的Linux多线程求和程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define ARRAY_SIZE 1000000
int array[ARRAY_SIZE];
long sum = 0;
pthread_mutex_t mutex_sum = PTHREAD_MUTEX_INITIALIZER;
void *thread_sum(void *arg) {
long thread_id = (long) arg;
long start = thread_id * ARRAY_SIZE / NUM_THREADS;
long end = (thread_id + 1) * ARRAY_SIZE / NUM_THREADS;
long local_sum = 0;
for (long i = start; i < end; i++) {
local_sum += array[i];
}
pthread_mutex_lock(&mutex_sum);
sum += local_sum;
pthread_mutex_unlock(&mutex_sum);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
for (long i = 0; i < ARRAY_SIZE; i++) {
array[i] = rand() % 100;
}
for (long i = 0; i < NUM_THREADS; i++) {
pthread_create(&threads[i], NULL, thread_sum, (void *) i);
}
for (long i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
printf("Sum: %ld\n", sum);
pthread_exit(NULL);
}
```
该程序使用4个线程并行计算一个大小为1000000的数组中所有元素的和。每个线程只计算数组的一部分,并将其局部和加到全局和中。由于多个线程同时修改全局和,需要使用互斥锁来保护它。最后,主线程打印出全局和的值。
注意,该程序中的线程数量和数组大小都是硬编码的,实际应用中需要根据具体情况进行调整。
相关推荐
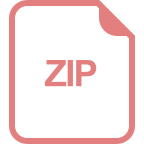
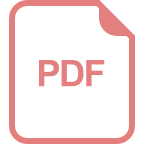
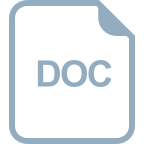
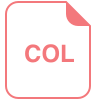
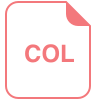
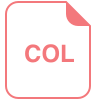
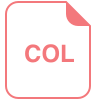
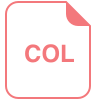







