使用JDK8时间日期类,模拟用户发货时间计算功能 //如果用户是当天下午3点前下单,则第二天下午3点前发货 //如果用户是当天下午3点及之后下单,则第三天下午3点前发货 //如果发货当天是周日,则在原发货时间基础性再加1天 //根据用户下单时间(自己构建),给出预计发货时间
时间: 2024-04-14 22:27:30 浏览: 178
可以使用Java 8的时间日期类来模拟用户发货时间计算功能。下面是一个示例代码:
```java
import java.time.DayOfWeek;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.temporal.ChronoUnit;
public class DeliveryTimeCalculator {
public static LocalDateTime calculateDeliveryTime(LocalDateTime orderTime) {
LocalTime cutoffTime = LocalTime.of(15, 0); // 下午3点为截止时间
// 如果用户是当天下午3点前下单,则第二天下午3点前发货
if (orderTime.toLocalTime().isBefore(cutoffTime)) {
return orderTime.plus(1, ChronoUnit.DAYS).with(cutoffTime);
}
// 如果用户是当天下午3点及之后下单,则第三天下午3点前发货
LocalDateTime deliveryTime = orderTime.plus(2, ChronoUnit.DAYS).with(cutoffTime);
// 如果发货当天是周日,则在原发货时间基础上再加1天
if (deliveryTime.getDayOfWeek() == DayOfWeek.SUNDAY) {
deliveryTime = deliveryTime.plus(1, ChronoUnit.DAYS);
}
return deliveryTime;
}
public static void main(String[] args) {
// 假设用户下单时间为2022-01-01 14:30:00
LocalDateTime orderTime = LocalDateTime.of(2022, 1, 1, 14, 30, 0);
LocalDateTime deliveryTime = calculateDeliveryTime(orderTime);
System.out.println("预计发货时间:" + deliveryTime);
}
}
```
在上面的示例中,我们定义了一个`calculateDeliveryTime`方法,它接收用户下单时间作为参数,并返回预计的发货时间。根据题目要求,我们首先检查用户下单时间是否在当天下午3点前,如果是,则发货时间为第二天下午3点前;否则,发货时间为第三天下午3点前。然后,我们检查发货时间是否为周日,如果是,则在原发货时间的基础上再加1天。
在`main`方法中,我们可以通过调用`calculateDeliveryTime`方法来获取预计的发货时间并输出结果。在示例中,假设用户下单时间为2022-01-01 14:30:00。
请注意,这只是一个示例代码,你可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
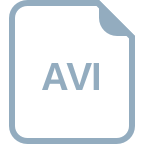
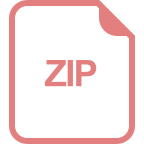
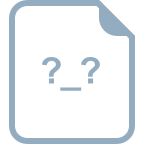
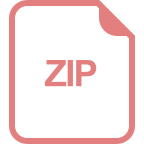
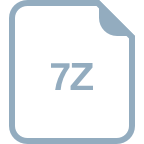
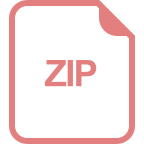
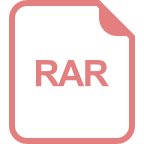
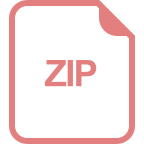
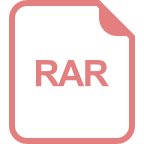
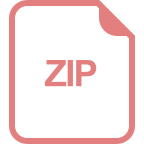
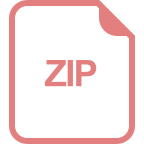
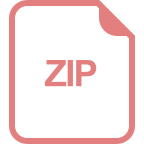
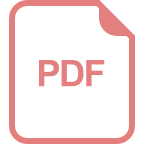
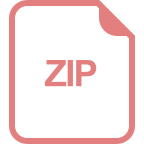
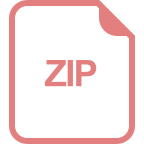