结构体数组 排序
时间: 2023-07-20 14:10:12 浏览: 57
结构体数组的排序可以使用C++中的标准库算法sort()函数。sort()函数需要传入两个迭代器,表示排序的起始位置和结束位置。对于结构体数组,我们需要自定义排序函数,以告诉sort()函数按照哪个字段进行排序。
例如,我们有一个存储学生信息的结构体数组:
```
struct student {
char name[20];
int age;
float grade;
} class[50];
```
我们可以按照学生的成绩进行排序,代码如下:
```
bool cmp(const student &a, const student &b) {
return a.grade > b.grade;
}
sort(class, class + 50, cmp);
```
在上面的代码中,我们自定义了一个排序函数cmp,它接受两个学生结构体的引用作为参数,并根据成绩字段进行比较。最后,我们将排序结果存储在原数组中。如果需要按照其他字段进行排序,只需要修改cmp函数的比较方式即可。
相关问题
结构体数组排序
可以使用C++中的STL库中的sort函数对结构体数组进行排序,具体步骤如下:
1. 定义结构体类型和结构体数组:
```C++
struct Student {
string name;
int score;
};
Student stu[5] = {{"Tom", 90}, {"Jack", 80}, {"Lily", 95}, {"Bob", 70}, {"Lucy", 85}};
```
2. 定义比较函数:
```C++
bool cmp(Student a, Student b) {
return a.score > b.score; // 按照分数从高到低排序
}
```
3. 使用sort函数进行排序:
```C++
sort(stu, stu + 5, cmp); // 对stu数组进行排序,使用cmp函数作为比较函数
```
4. 输出排序结果:
```C++
for (int i = 0; i < 5; i++) {
cout << stu[i].name << " " << stu[i].score << endl;
}
```
完整代码如下:
```C++
#include <iostream>
#include <algorithm>
using namespace std;
struct Student {
string name;
int score;
};
bool cmp(Student a, Student b) {
return a.score > b.score; // 按照分数从高到低排序
}
int main() {
Student stu[5] = {{"Tom", 90}, {"Jack", 80}, {"Lily", 95}, {"Bob", 70}, {"Lucy", 85}};
sort(stu, stu + 5, cmp); // 对stu数组进行排序,使用cmp函数作为比较函数
for (int i = 0; i < 5; i++) {
cout << stu[i].name << " " << stu[i].score << endl;
}
return 0;
}
```
输出结果为:
```
Lily 95
Tom 90
Lucy 85
Jack 80
Bob 70
```
c++结构体数组排序
在C语言中,可以通过重载"<"比较运算符或者使用内置的比较函数来对结构体数组进行排序。这里提供了三种不同的方法来实现结构体数组的排序。
第一种方法是在结构体内部重载"<"比较运算符。我们可以定义一个结构体node,其中包含两个整型成员x和y。在结构体内部,我们重载"<"比较运算符,通过比较x和y的值来决定结构体的排序方式。然后我们声明一个结构体数组a,并将其元素按照我们定义的排序规则进行排序。这种方法可以避免使用sort函数,直接在结构体内部进行排序。
第二种方法是使用内置的比较函数less<type>或greater<type>。我们可以使用sort函数对结构体数组a进行排序,通过传入less<int>()或者greater<int>()来指定排序的顺序,从小到大或者从大到小。这种方法可以简化代码,但需要使用sort函数。
第三种方法是通过重载"<"比较运算符来规定sort排序方法。与第一种方法类似,我们定义一个结构体node,并在结构体外部定义友元函数operator<来重载"<"比较运算符。在函数内部,我们比较两个结构体的x和y值,并根据比较结果决定排序方式。然后我们声明一个结构体数组a,并调用sort函数对数组进行排序。
总之,在C语言中,我们可以通过重载"<"比较运算符或者使用内置的比较函数来对结构体数组进行排序。具体的方法取决于个人的需求和偏好。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C++结构体如何排序](https://blog.csdn.net/Ivoritow/article/details/129231105)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
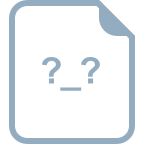
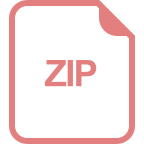
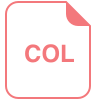
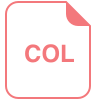
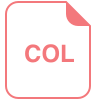
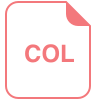







