for i,row in enumerate(sheet.get_rows())
时间: 2024-04-11 15:29:43 浏览: 154
这段代码使用了Python中的enumerate函数和sheet对象的get_rows方法来遍历Excel表格中的行并返回行索引和对应的行数据。
在这个例子中,sheet表示一个Excel表格对象,get_rows方法用于获取所有行的数据。enumerate函数则用于将获取到的行数据和对应的索引配对,然后通过for循环逐个处理。
您可以继续提出您的问题或补充代码,我会尽力帮助您。
相关问题
def show_sheet(self, sheet_name): self.textbox.tag_configure("left", justify="left") sheet = self.workbook[sheet_name] rows = sheet.max_row # 清空文本框 self.textbox.delete(1.0, tk.END) # 添加表名并设置居中标签 self.textbox.insert(tk.END, sheet_name + ":\n", "center") # 显示工作表内容,并在相应数据后面添加下拉输入框 for row in sheet.iter_rows(values_only=True): for i, cell in enumerate(row): line = str(cell) + "\t" if i == 0: # 在第一列数据后面添加下拉输入框 combobox = tk.ttk.Combobox(self.textbox, values=["下拉选项1", "下拉选项2", "下拉选项3"]) combobox.pack(side=tk.TOP, padx=10, pady=5) self.textbox.window_create(tk.END, window=combobox) self.textbox.insert(tk.END, line, "left") self.textbox.insert(tk.END, "\n") # 设置居中标签的样式 self.textbox.tag_configure("center", justify="center", font=("Arial", 14, "bold"))根據這個函數在寫一個函數,將這個函數生成文本框中的數據内容和下拉輸入框的内容按列寫入一個excel中,在寫入前要判斷文本框中是否存在内容和下拉輸入框是否有值,如果沒有就提醒
您可以使用openpyxl模块来实现将文本框中的内容和下拉输入框的值按列写入Excel的功能。可以先定义一个函数,将文本框中的数据和下拉框的值读取出来,并存储到一个二维数组中,再使用openpyxl模块将其写入Excel文件中。下面是一个可能的实现方式:
```python
import openpyxl
def save_to_excel(sheet_name, textbox):
sheet_data = [] # 存储表格数据的二维数组
for line in textbox.get("1.0", "end").split("\n"):
line_data = []
if line.strip() == "": # 跳过空行
continue
for i, cell in enumerate(line.split("\t")):
if i == 0: # 第一列为下拉框的值,需要特殊处理
if cell.startswith("Combobox("): # 如果是下拉框,则取其值
cell = cell.split("(")[1].split(")")[0]
else: # 否则为空值
cell = ""
line_data.append(cell)
sheet_data.append(line_data)
if len(sheet_data) == 0: # 如果表格中没有数据,则提醒用户
messagebox.showwarning("提示", "表格中没有数据,无法保存!")
return
wb = openpyxl.Workbook()
ws = wb.active
ws.title = sheet_name
for row_data in sheet_data:
ws.append(row_data)
wb.save("output.xlsx")
messagebox.showinfo("提示", "保存成功!")
```
这个函数需要两个参数,`sheet_name`表示要保存的工作表名称,`textbox`表示要读取数据的文本框。函数先将文本框中的内容按行读取,并将每行的数据按制表符分割成一个列表。然后对于每个列表中的元素,如果是第一列,则需要特殊处理,因为第一列是下拉框的值。如果是下拉框,则取其值,否则为空值。最后将每行的数据存储到一个二维数组中。
如果表格中没有数据,则弹出提示框提醒用户。否则,创建一个新的Excel文件并写入数据。最后弹出提示框告诉用户保存成功。
# 创建工作簿和工作表 workbook = openpyxl.Workbook() sheet = workbook.active # 从文本框和下拉框中获取数据,并按列写入Excel文件 rows = self.textbox.get("1.0", "end").split("\n") for row_index, row in enumerate(rows): if not row.strip(): continue cells = row.split("\t") for cell_index, cell in enumerate(cells): if cell_index > sheet.max_column - 1: sheet.cell(row=row_index + 1, column=cell_index + 1, value=cell) else: sheet.cell(row=row_index + 1, column=cell_index + 1).value = cell for widget_index, widget in enumerate(self.textbox.winfo_children()): if isinstance(widget, tk.ttk.Combobox) and widget_index == row_index: sheet.cell(row=row_index + 1, column=len(cells) + 1, value=widget.get()) # 保存Excel文件 workbook.save(r"D:\點檢系統存放資料夾\點檢明細\1.xlsx")修改這段代碼中如果沒有日志框數據就跳過寫入
可以在循环内部添加一个判断,如果当前行为空行,则跳过写入Excel文件的操作。修改后的代码如下:
```
workbook = openpyxl.Workbook()
sheet = workbook.active
rows = self.textbox.get("1.0", "end").split("\n")
for row_index, row in enumerate(rows):
if not row.strip():
continue # 当前行为空行,跳过写入Excel文件的操作
cells = row.split("\t")
for cell_index, cell in enumerate(cells):
if cell_index > sheet.max_column - 1:
sheet.cell(row=row_index + 1, column=cell_index + 1, value=cell)
else:
sheet.cell(row=row_index + 1, column=cell_index + 1).value = cell
for widget_index, widget in enumerate(self.textbox.winfo_children()):
if isinstance(widget, tk.ttk.Combobox) and widget_index == row_index:
sheet.cell(row=row_index + 1, column=len(cells) + 1, value=widget.get())
workbook.save(r"D:\點檢系統存放資料夾\點檢明細\1.xlsx")
```
阅读全文
相关推荐
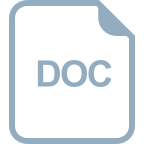
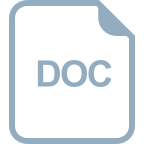
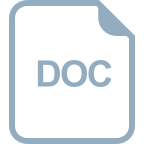

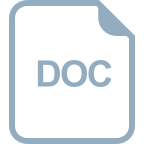
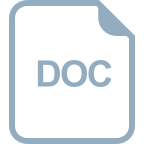
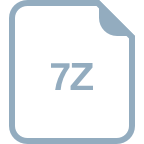
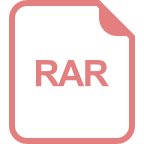
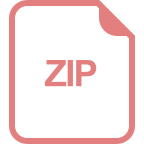
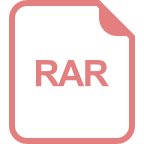
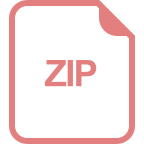
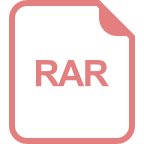
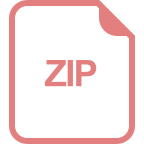
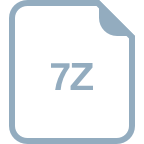
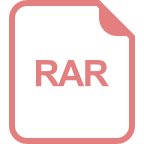
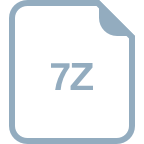
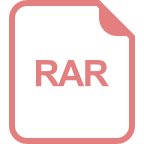