vue tabbar底部导航栏
时间: 2023-03-24 21:03:38 浏览: 150
Vue tabbar底部导航栏是一种常见的UI组件,用于在移动端或者Web端展示多个页面或者功能入口。它通常由多个按钮组成,每个按钮代表一个页面或者功能,用户可以通过点击按钮来切换页面或者进入相应的功能。Vue tabbar底部导航栏可以通过Vue组件库或者自定义组件来实现。
相关问题
Vue3实现TabBar底部导航栏灵活组件
Vue3实现TabBar底部导航栏灵活组件的步骤如下:
1. 创建一个Vue组件,并定义需要的props属性,比如标签页列表、当前选中的标签页等。
2. 在组件中使用Vue3的Composition API,定义需要的状态和方法,比如当前选中的标签页索引、切换标签页的方法等。
3. 在组件的template中使用v-for指令渲染标签页列表,并使用v-bind指令动态绑定类名和样式。
4. 在组件中使用v-on指令绑定click事件,触发切换标签页的方法。
5. 在父组件中引用TabBar组件,并传入需要的props属性。
6. 在父组件中可以使用slot插槽,自定义每个标签页的内容。
7. 可以根据不同的需求,自定义TabBar组件的样式和动画效果。
示例代码如下:
```html
<template>
<div class="tab-bar">
<div v-for="(item, index) in tabs" :key="index"
:class="{ 'tab': true, 'active': currentIndex === index }"
@click="switchTab(index)">
{{ item.label }}
</div>
</div>
<div class="tab-content">
<slot :name="tabs[currentIndex].name"></slot>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'TabBar',
props: {
tabs: {
type: Array,
required: true
},
defaultIndex: {
type: Number,
default: 0
}
},
setup(props) {
const currentIndex = ref(props.defaultIndex);
const switchTab = (index) => {
currentIndex.value = index;
};
return {
currentIndex,
switchTab
};
}
};
</script>
<style>
.tab-bar {
display: flex;
justify-content: space-around;
align-items: center;
height: 50px;
border-top: 1px solid #ccc;
background-color: #fff;
}
.tab {
display: flex;
justify-content: center;
align-items: center;
width: 20%;
height: 100%;
font-size: 14px;
color: #333;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
.active {
color: #1890ff;
}
.tab-content {
padding: 20px;
background-color: #f5f5f5;
}
</style>
```
vue3实现TabBar底部导航栏灵活组件
Vue3中可以通过组合API和自定义组件等方式实现灵活的TabBar底部导航栏组件,以下是一个简单的实现示例:
1. 首先定义一个TabBarItem组件,用于渲染每个底部导航栏项的内容,包括图标和文本等。
```javascript
<template>
<div class="tab-bar-item" :class="{ active: active }" @click="handleClick">
<span class="tab-bar-icon">{{ icon }}</span>
<span class="tab-bar-text">{{ text }}</span>
</div>
</template>
<script>
export default {
props: {
icon: {
type: String,
required: true
},
text: {
type: String,
required: true
},
active: {
type: Boolean,
default: false
}
},
emits: ['change'],
methods: {
handleClick() {
this.$emit('change');
}
}
};
</script>
```
2. 然后定义一个TabBar组件,用于渲染整个底部导航栏,同时管理和切换每个TabBarItem的状态。
```javascript
<template>
<div class="tab-bar">
<tab-bar-item
v-for="(item, index) in tabs"
:key="index"
:icon="item.icon"
:text="item.text"
:active="index === activeIndex"
@change="handleChange(index)"
/>
</div>
</template>
<script>
import TabBarItem from './TabBarItem.vue';
export default {
components: {
TabBarItem
},
props: {
tabs: {
type: Array,
required: true
},
defaultIndex: {
type: Number,
default: 0
}
},
emits: ['change'],
data() {
return {
activeIndex: this.defaultIndex
};
},
methods: {
handleChange(index) {
if (index !== this.activeIndex) {
this.activeIndex = index;
this.$emit('change', index);
}
}
}
};
</script>
```
3. 最后在父组件中使用TabBar组件,传入需要显示的底部导航栏项和对应的内容组件,实现灵活的底部导航栏功能。
```javascript
<template>
<div class="app">
<router-view />
<tab-bar :tabs="tabs" @change="handleChange" />
</div>
</template>
<script>
import TabBar from './TabBar.vue';
import Home from './Home.vue';
import About from './About.vue';
export default {
components: {
TabBar,
Home,
About
},
data() {
return {
tabs: [
{
icon: 'home',
text: 'Home',
component: Home
},
{
icon: 'about',
text: 'About',
component: About
}
],
activeComponent: null
};
},
methods: {
handleChange(index) {
this.activeComponent = this.tabs[index].component;
}
}
};
</script>
```
在以上代码中,父组件通过监听TabBar组件的change事件,动态切换显示对应的内容组件,实现了灵活的底部导航栏功能。
阅读全文
相关推荐
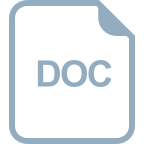
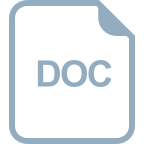
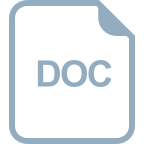
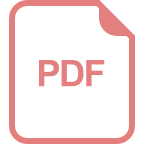





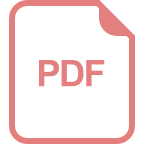






