springboot redis yml 配置文件‘’
时间: 2023-06-12 08:05:02 浏览: 181
以下是一个简单的 Spring Boot + Redis 的配置文件示例,包括 Redis 主机名、端口号、密码等配置:
```
spring:
redis:
host: localhost
port: 6379
password: yourpassword
timeout: 3000 #连接超时时间,单位ms
jedis:
pool:
max-active: 8 #连接池最大连接数
max-wait: -1 #连接池最大阻塞等待时间,单位ms,小于零则表示无限等待
max-idle: 8 #连接池中的最大空闲连接
min-idle: 0 #连接池中的最小空闲连接
lettuce:
pool:
max-active: 8 #连接池最大连接数
max-wait: -1 #连接池最大阻塞等待时间,单位ms,小于零则表示无限等待
max-idle: 8 #连接池中的最大空闲连接
min-idle: 0 #连接池中的最小空闲连接
```
其中,`host`、`port`、`password` 分别是 Redis 主机名、端口号和密码,`timeout` 是连接超时时间,单位是毫秒。`jedis` 和 `lettuce` 是 Redis 的两种不同的客户端实现,可以根据需要选择其中一种,并配置连接池的参数。
相关问题
springboot redis lettuce 配置文件
在Spring Boot中,你可以在`application.properties`或`application.yml`配置文件中配置Redis的连接信息。以下是一个示例的配置:
在`application.properties`中的配置示例:
```properties
# Redis连接信息
spring.redis.host=your-redis-host
spring.redis.port=your-redis-port
spring.redis.password=your-redis-password
# Lettuce连接池配置
spring.redis.lettuce.pool.max-active=10
spring.redis.lettuce.pool.max-idle=5
spring.redis.lettuce.pool.min-idle=2
spring.redis.lettuce.pool.max-wait=3000
```
在`application.yml`中的配置示例:
```yaml
# Redis连接信息
spring:
redis:
host: your-redis-host
port: your-redis-port
password: your-redis-password
# Lettuce连接池配置
spring:
redis:
lettuce:
pool:
max-active: 10
max-idle: 5
min-idle: 2
max-wait: 3000
```
你可以根据实际情况修改以上配置信息,将`your-redis-host`、`your-redis-port`和`your-redis-password`替换为实际的Redis主机名、端口号和密码。此外,你还可以根据需要调整Lettuce连接池的配置参数。完成配置后,Spring Boot应用程序将使用Lettuce连接到Redis服务器。
springboot redis集群配置文件
在Spring Boot中配置Redis集群需要进行以下步骤:
1. 首先,确保你已经在你的项目中添加了Redis的依赖,可以通过在pom.xml文件中添加以下依赖来实现:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 在你的application.properties或application.yml文件中添加Redis相关的配置。以下是一个示例配置:
```properties
spring.redis.cluster.nodes=127.0.0.1:7001,127.0.0.1:7002,127.0.0.1:7003
spring.redis.password=yourpassword
spring.redis.timeout=3000
spring.redis.cluster.max-redirects=3
```
或者在application.yml文件中:
```yaml
spring:
redis:
cluster:
nodes: 127.0.0.1:7001,127.0.0.1:7002,127.0.0.1:7003
password: yourpassword
timeout: 3000
cluster.max-redirects: 3
```
请注意,`spring.redis.cluster.nodes`属性是Redis集群中所有节点的列表,每个节点由主机和端口组成。`spring.redis.password`属性是可选的,如果你的Redis集群有密码,则需要提供密码。`spring.redis.timeout`属性是连接超时时间(以毫秒为单位),`spring.redis.cluster.max-redirects`属性是当连接到一个节点失败时,最大重定向次数。
3. 创建一个RedisTemplate Bean来使用Redis集群。你可以在你的配置类中添加以下代码:
```java
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
return new LettuceConnectionFactory(new RedisClusterConfiguration());
}
@Bean
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<Object, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
return redisTemplate;
}
}
```
这里使用了Lettuce作为Redis客户端的连接工厂,`RedisClusterConfiguration`用于配置Redis集群的连接信息。
现在,你可以在你的应用程序中使用`RedisTemplate`来访问Redis集群了。
希望这可以帮助到你!如有其他问题,请随时提问。
阅读全文
相关推荐
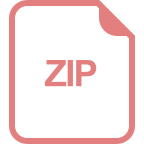
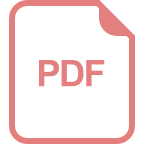
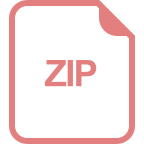













