C语言实现加减乘除随机出题错题重做功能
时间: 2023-07-18 08:34:40 浏览: 125
好的,这是一个比较复杂的问题,我会尽可能详细地回答。首先,为了实现加减乘除的随机出题,我们需要使用随机数生成器来生成两个操作数和一个运算符。C语言中可以使用rand()函数来生成随机数,但是需要先调用srand()函数设置随机数种子。
下面是一个示例代码,可以随机生成包含加减乘除四种运算的数学题目:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
int operand1, operand2, result;
char operator;
srand(time(NULL)); // 设置随机数种子为当前时间
// 生成随机数和运算符
operand1 = rand() % 100;
operand2 = rand() % 100;
switch (rand() % 4) {
case 0: operator = '+'; result = operand1 + operand2; break;
case 1: operator = '-'; result = operand1 - operand2; break;
case 2: operator = '*'; result = operand1 * operand2; break;
case 3: operator = '/'; result = operand1 / operand2; break;
}
// 输出题目
printf("%d %c %d = ?\n", operand1, operator, operand2);
return 0;
}
```
接下来是实现错题重做功能的部分。我们可以使用一个数组来保存错题的题目和答案,然后在程序结束之前输出这些错题,让用户重新回答。
下面是一个简单的示例代码,可以实现记录错题和重做错题的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_WRONG_ANSWERS 10
struct Question {
int operand1, operand2, answer;
char operator;
};
int main() {
int num_wrong_answers = 0;
struct Question wrong_answers[MAX_WRONG_ANSWERS];
srand(time(NULL)); // 设置随机数种子为当前时间
// 循环出题
while (num_wrong_answers < MAX_WRONG_ANSWERS) {
int operand1 = rand() % 100;
int operand2 = rand() % 100;
int answer;
char operator;
switch (rand() % 4) {
case 0: operator = '+'; answer = operand1 + operand2; break;
case 1: operator = '-'; answer = operand1 - operand2; break;
case 2: operator = '*'; answer = operand1 * operand2; break;
case 3: operator = '/'; answer = operand1 / operand2; break;
}
// 输出题目
printf("%d %c %d = ?\n", operand1, operator, operand2);
// 获取用户输入
int user_answer;
scanf("%d", &user_answer);
// 检查用户答案是否正确,如果不正确则记录下来
if (user_answer != answer) {
struct Question wrong_answer = {operand1, operand2, answer, operator};
wrong_answers[num_wrong_answers++] = wrong_answer;
printf("Wrong answer. The correct answer is %d.\n", answer);
} else {
printf("Correct!\n");
}
}
// 输出错题并让用户重做
printf("You have answered %d questions incorrectly. Here are the wrong answers:\n", num_wrong_answers);
for (int i = 0; i < num_wrong_answers; i++) {
printf("%d %c %d = %d\n", wrong_answers[i].operand1, wrong_answers[i].operator, wrong_answers[i].operand2, wrong_answers[i].answer);
printf("Please answer again: ");
int user_answer;
scanf("%d", &user_answer);
if (user_answer != wrong_answers[i].answer) {
printf("Wrong answer again. The correct answer is %d.\n", wrong_answers[i].answer);
} else {
printf("Correct!\n");
}
}
return 0;
}
```
以上是一个简单的实现,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐

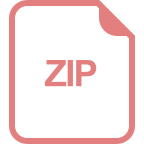
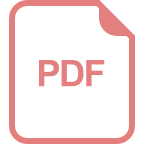
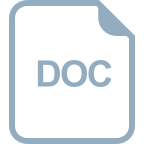

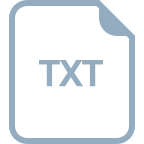
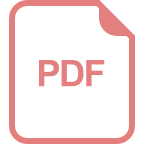
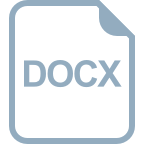
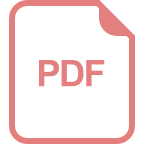