linux生产者消费者源程序代码
时间: 2023-11-21 12:08:30 浏览: 68
以下是一个简单的 Linux 生产者消费者模型的源代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int count = 0;
int in = 0;
int out = 0;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
pthread_cond_t empty = PTHREAD_COND_INITIALIZER;
void *producer(void *arg)
{
int i;
for (i = 0; i < 20; i++) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
pthread_cond_wait(&empty, &mutex);
}
buffer[in] = i;
in = (in + 1) % BUFFER_SIZE;
count++;
printf("Producer: Produced %d\n", i);
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
sleep(rand() % 3);
}
pthread_exit(NULL);
}
void *consumer(void *arg)
{
int i;
for (i = 0; i < 20; i++) {
pthread_mutex_lock(&mutex);
while (count == 0) {
pthread_cond_wait(&full, &mutex);
}
int consumed = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
printf("Consumer: Consumed %d\n", consumed);
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
sleep(rand() % 3);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[])
{
pthread_t producer_thread, consumer_thread;
srand(time(NULL));
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
```
这个程序使用了 pthread 库中的互斥锁和条件变量来实现同步。生产者线程和消费者线程通过互斥锁来保护共享缓冲区,通过条件变量来协调彼此之间的行为。当缓冲区已满时,生产者线程会等待条件变量 empty,直到缓冲区有空间可用;当缓冲区为空时,消费者线程会等待条件变量 full,直到缓冲区有数据可用。
阅读全文
相关推荐





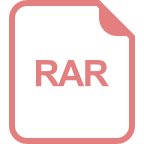
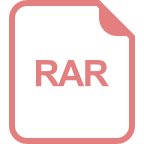

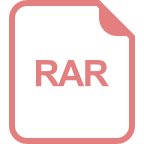
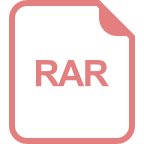



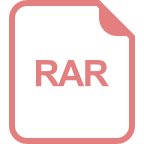
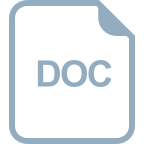




