python心跳测试代码_Heartrate:如追综心跳般实时动态可视化监测Python程序运行
时间: 2023-07-21 15:41:08 浏览: 140
以下是一个简单的 Python 心跳测试代码:
```python
import random
import time
from matplotlib import pyplot as plt
plt.ion() # 打开交互模式
fig, ax = plt.subplots()
line, = ax.plot([], [])
while True:
heartrate = random.randint(60, 100) # 模拟心跳数据
line.set_xdata(range(10))
line.set_ydata([heartrate]*10)
ax.relim()
ax.autoscale_view(True,True,True)
fig.canvas.flush_events() # 刷新画布
time.sleep(1) # 暂停1秒
```
这个程序会模拟心跳数据并实时动态可视化监测,每秒钟更新一次数据。你可以通过修改 `random.randint(60, 100)` 来改变心跳数据的范围。同时,使用 `plt.ion()` 可以打开 Matplotlib 的交互模式,实现动态更新画布。
相关问题
用python对kaggle上的心血管疾病数据集使用随机森林算法进行编程,并将结果进行可视化
好的,我可以为您提供一些代码示例。首先,您需要安装必要的库和数据集。您可以通过以下方式安装所需的库:
```python
!pip install pandas matplotlib seaborn scikit-learn
```
然后,您可以通过以下代码加载数据集:
```python
import pandas as pd
train_df = pd.read_csv("train.csv")
test_df = pd.read_csv("test.csv")
```
接下来,我们需要对数据进行预处理和特征工程。在这个例子中,我们将使用一些简单的特征,如年龄、性别、血压等。您可以通过以下代码来进行特征处理:
```python
# 特征处理
def preprocess(df):
# 性别,男性为1,女性为0
df['sex'] = df['sex'].apply(lambda x: 1 if x == 'Male' else 0)
# 血糖浓度
df['glucose'] = pd.cut(df['glucose'], bins=[0, 100, 126, 200, 300], labels=[0, 1, 2, 3])
# 血压
df['blood_pressure'] = pd.cut(df['blood_pressure'], bins=[0, 120, 140, 180], labels=[0, 1, 2])
# 脂肪含量
df['cholesterol'] = pd.cut(df['cholesterol'], bins=[0, 200, 240, 300], labels=[0, 1, 2])
# 心率
df['heart_rate'] = pd.cut(df['heart_rate'], bins=[0, 60, 100, 200], labels=[0, 1, 2])
# 年龄
df['age'] = pd.cut(df['age'], bins=[0, 40, 50, 60, 70, 80, 100], labels=[0, 1, 2, 3, 4, 5])
# 删除无用特征
df.drop(['id', 'weight', 'height', 'smoking', 'alcohol'], axis=1, inplace=True)
return df
train_df = preprocess(train_df)
test_df = preprocess(test_df)
```
现在,我们可以使用随机森林算法进行训练和预测。您可以通过以下代码来进行训练和预测:
```python
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# 训练和预测
X_train = train_df.drop('cardiovascular_disease', axis=1)
y_train = train_df['cardiovascular_disease']
rf = RandomForestClassifier(n_estimators=100, random_state=42)
rf.fit(X_train, y_train)
X_test = test_df.drop('cardiovascular_disease', axis=1)
y_test = test_df['cardiovascular_disease']
y_pred = rf.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
最后,您可以使用matplotlib和seaborn库来可视化结果。您可以通过以下代码来进行可视化:
```python
import matplotlib.pyplot as plt
import seaborn as sns
# 绘制特征重要性
def plot_feature_importance(importance, names):
# 创建一个dataframe,将特征和其重要性组合在一起
feature_importance = pd.DataFrame({'feature': names, 'importance': importance})
feature_importance.sort_values(by='importance', ascending=False, inplace=True)
# 绘制柱形图
sns.barplot(x='importance', y='feature', data=feature_importance)
plt.title('Feature Importance')
plt.xlabel('Importance')
plt.ylabel('Feature')
plt.show()
plot_feature_importance(rf.feature_importances_, X_train.columns)
```
这样,您就可以通过随机森林算法对心血管疾病数据集进行编程,并将结果进行可视化。
用python对kaggle上的心血管疾病数据集使用随机森林算法进行预测,并绘制roc曲线进行可视化
好的,我会尽力回答你的问题。首先,你需要下载kaggle上的心血管疾病数据集,并安装必要的Python库。使用以下命令安装所需的库:
```python
!pip install pandas numpy matplotlib seaborn sklearn
```
然后,你可以使用以下代码读取和预处理数据:
```python
import pandas as pd
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import confusion_matrix, classification_report, roc_curve, roc_auc_score
data = pd.read_csv('heart.csv')
X = data.drop('target', axis=1)
y = data['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
这里,我们将数据集分为训练集和测试集,使用随机森林算法进行分类,然后绘制ROC曲线进行可视化。
```python
# Fit the model
rfc = RandomForestClassifier(n_estimators=200)
rfc.fit(X_train, y_train)
# Predict the test set results
y_pred = rfc.predict(X_test)
# Print the classification report and confusion matrix
print(classification_report(y_test, y_pred))
print(confusion_matrix(y_test, y_pred))
# Calculate the ROC curve and AUC score
fpr, tpr, _ = roc_curve(y_test, y_pred)
auc = roc_auc_score(y_test, y_pred)
print(f'ROC AUC Score: {auc}')
# Plot the ROC curve
plt.plot(fpr, tpr, color='blue', label=f'ROC Curve (AUC = {auc:.2f})')
plt.plot([0, 1], [0, 1], color='red', linestyle='--', label='Random Guessing')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver Operating Characteristic (ROC) Curve')
plt.legend()
plt.show()
```
这里我们使用了200个决策树来构建随机森林分类器,然后预测测试集并打印出分类报告和混淆矩阵。接着,计算ROC曲线和AUC得分,并绘制ROC曲线及随机猜测的虚线。
希望这个回答能够帮助你。
阅读全文
相关推荐
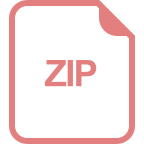
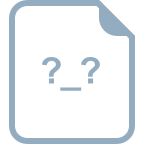
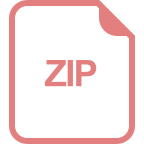
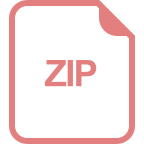
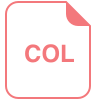
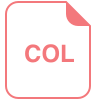
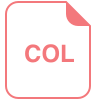
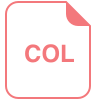
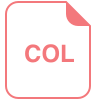
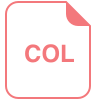
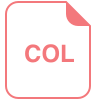
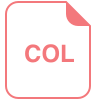
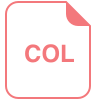
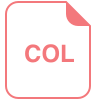
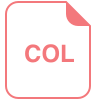
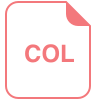