mybatisplus collection一对多分页问题
时间: 2023-06-28 21:06:12 浏览: 83
MybatisPlus 的 collection 一对多查询可以使用子查询或者关联查询两种方式实现,其中关联查询方式最常用。对于一对多关联查询的分页问题,可以按照以下步骤实现:
1. 定义一个包含分页参数的 DTO 类,例如:
```java
public class MyDto {
private Integer pageNum;
private Integer pageSize;
// 其他查询条件
// ...
}
```
2. 在 Mapper 接口中定义一个方法,例如:
```java
List<MyEntity> selectMyEntityList(@Param("dto") MyDto dto);
```
3. 在 Mapper.xml 文件中编写关联查询 SQL,例如:
```xml
<select id="selectMyEntityList" resultMap="myEntityResultMap">
select me.*, mc.*
from my_entity me
left join my_child mc on me.id = mc.entity_id
<where>
<!-- 根据查询条件动态拼接 SQL -->
<if test="dto.xxx != null">
and me.xxx = #{dto.xxx}
</if>
<!-- 其他查询条件 -->
<!-- ... -->
</where>
order by me.create_time desc
</select>
```
4. 在 Service 中调用 Mapper 方法并进行分页处理,例如:
```java
public Page<MyEntity> selectMyEntityPage(MyDto dto) {
// 设置分页参数
Page<MyEntity> page = new Page<>(dto.getPageNum(), dto.getPageSize());
// 执行 MybatisPlus 的分页查询
IPage<MyEntity> iPage = myMapper.selectMyEntityList(page, dto);
// 将 IPage 转换为 Page 并返回
return new Page<>(iPage.getCurrent(), iPage.getSize(), iPage.getTotal()).setRecords(iPage.getRecords());
}
```
5. 在 Controller 中接收前端传递的分页参数并调用 Service 方法,例如:
```java
@GetMapping("/myEntities")
public Result<List<MyEntity>> getMyEntityList(MyDto dto) {
Page<MyEntity> page = myService.selectMyEntityPage(dto);
return Result.success(page.getRecords()).setPageInfo(page);
}
```
以上就是使用 MybatisPlus 实现一对多关联查询的分页处理的基本步骤。需要注意的是,如果是使用子查询方式实现一对多关联查询,则需要将子查询的结果集转换为 List 并进行分页处理。
相关推荐
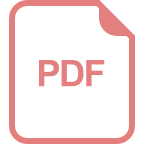
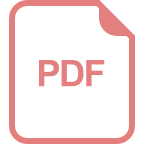














