神经网络模型python代码
时间: 2023-10-13 14:13:30 浏览: 40
以下是一个简单的神经网络模型的Python代码示例:
```python
import numpy as np
# 定义神经网络类
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 随机初始化权重和偏置
self.weights1 = np.random.randn(self.input_size, self.hidden_size)
self.bias1 = np.random.randn(self.hidden_size)
self.weights2 = np.random.randn(self.hidden_size, self.output_size)
self.bias2 = np.random.randn(self.output_size)
# 定义 sigmoid 激活函数
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
# 定义神经网络的前向传播
def forward(self, x):
z1 = np.dot(x, self.weights1) + self.bias1
a1 = self.sigmoid(z1)
z2 = np.dot(a1, self.weights2) + self.bias2
y = self.sigmoid(z2)
return y
# 测试代码
nn = NeuralNetwork(2, 3, 1)
x = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
for i in range(10000):
output = nn.forward(x)
loss = np.mean(np.square(output - y))
if i % 1000 == 0:
print("Loss:", loss)
# 反向传播
delta2 = (output - y) * output * (1 - output)
d_weights2 = np.dot(nn.sigmoid(nn.weights1), delta2)
delta1 = np.dot(delta2, nn.weights2.T) * nn.sigmoid(nn.weights1) * (1 - nn.sigmoid(nn.weights1))
d_weights1 = np.dot(x.T, delta1)
nn.weights1 -= 0.1 * d_weights1
nn.bias1 -= 0.1 * np.mean(delta1, axis=0)
nn.weights2 -= 0.1 * d_weights2
nn.bias2 -= 0.1 * np.mean(delta2, axis=0)
```
该模型使用 numpy 库来进行矩阵运算,实现了一个包含两个输入、三个隐藏单元和一个输出的神经网络。在训练过程中,使用反向传播算法来更新权重和偏置。
阅读全文
相关推荐
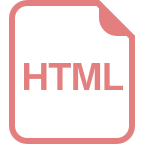
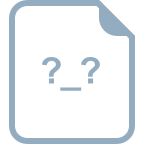
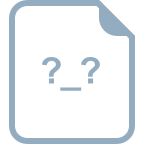
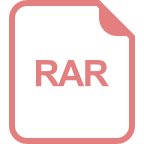
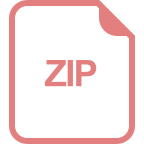
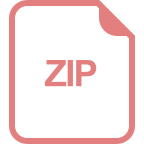
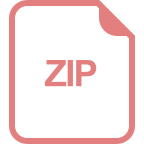