SpringBoot整合Redis
时间: 2023-03-21 11:01:33 浏览: 36
Spring Boot 集成 Redis 可以通过配置一些简单的配置来实现。
首先,需要在项目的 pom.xml 中添加对 Redis 的依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
其次,在 application.properties 或者 application.yml 中配置 Redis 连接信息:
```
# Redis 配置
spring.redis.host=localhost
spring.redis.port=6379
```
最后,通过 @Autowired 注入 RedisTemplate 来实现对 Redis 的操作。
```
@Autowired
private RedisTemplate<String, Object> redisTemplate;
```
综上所述,通过以上步骤,我们就可以在 Spring Boot 中集成 Redis 了。
相关问题
Springboot 整合 Redis
在Spring Boot中整合Redis,可以按照以下步骤进行操作:
1. 在pom.xml文件中添加Redis的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 在application.properties或application.yml文件中配置Redis连接信息:
```yaml
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
```
3. 创建一个需要使用Redis的服务类,注入RedisTemplate,并进行操作:
```java
@Service
public class MyService {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public void setValue(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Object getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
// 其他操作...
}
```
这样就完成了Spring Boot整合Redis的配置和使用。你可以在需要使用Redis的地方注入MyService,并调用其中的方法来操作Redis。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [SpringBoot整合Redis](https://blog.csdn.net/weixin_55772633/article/details/131945323)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
Springboot整合redis
Spring Boot整合Redis项目可以实现高效的缓存管理和数据存储。以下是实现步骤:
1. 添加Redis依赖
在pom.xml文件中添加Redis依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置Redis连接信息
在application.properties文件中添加Redis连接信息:
```
spring.redis.host=127...1
spring.redis.port=6379
spring.redis.password=
```
3. 创建RedisTemplate
在配置类中创建RedisTemplate:
```
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(factory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(new GenericJackson2JsonRedisSerializer());
return template;
}
}
```
4. 使用RedisTemplate操作Redis
在需要使用Redis的类中注入RedisTemplate,然后使用它操作Redis:
```
@Autowired
private RedisTemplate<String, Object> redisTemplate;
public void set(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Object get(String key) {
return redisTemplate.opsForValue().get(key);
}
```
以上就是Spring Boot整合Redis的基本步骤。
阅读全文
相关推荐
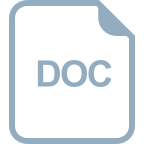
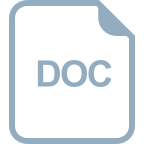
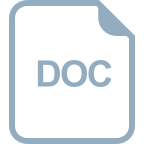
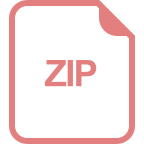

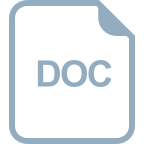
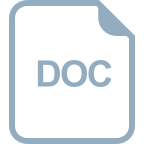
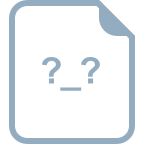
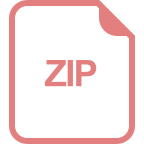
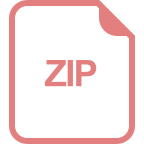
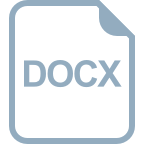
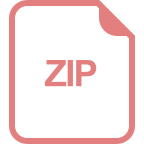
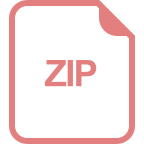
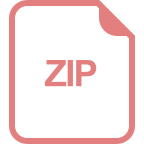