编程统计字符数组str中数字字符的个数
时间: 2024-01-28 07:05:53 浏览: 48
可以使用循环遍历字符串,判断每个字符是否为数字字符,如果是,则计数器加一。可以使用isdigit()函数来判断一个字符是否为数字字符。
以下是示例代码:
```c
#include <stdio.h>
#include <ctype.h> // 包含 isdigit() 函数的头文件
int main() {
char str[] = "abc123def456ghi";
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) { // 如果是数字字符
count++; // 计数器加一
}
}
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
输出结果为:
```
数字字符的个数为:6
```
相关问题
C语言编程统计字符数组str 中数字字符的个数
可以使用循环遍历字符数组,判断每个字符是否为数字字符,如果是则计数器加1。可以使用C标准库中的isdigit函数来判断字符是否为数字字符。
示例代码如下:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[] = "hello123world456";
int num_count = 0;
int i;
for (i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
num_count++;
}
}
printf("数字字符个数:%d\n", num_count);
return 0;
}
```
输出:
```
数字字符个数:6
```
编程统计字符数组str中数字字符的个数。
好的,以下是一个简单的C语言程序,可以统计字符数组str中数字字符的个数:
```c
#include <stdio.h>
int main() {
char str[] = "Hello1234World";
int count = 0;
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] >= '0' && str[i] <= '9') {
count++;
}
}
printf("数字字符的个数为:%d", count);
return 0;
}
```
程序输出:
```
数字字符的个数为:4
```
解释一下,这个程序首先定义了一个字符数组`str`,并且初始化为`"Hello1234World"`。接下来定义了一个计数变量`count`,用于记录数字字符的个数。然后使用for循环遍历字符数组`str`中的每个字符,如果字符是数字字符,就将`count`加1。最后输出`count`的值即可。
阅读全文
相关推荐
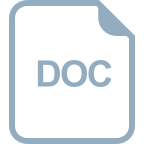












