用c++1.定义两个类:A和B,具有相同的变量x和问名的函数funsame);xsubAB继承A和B;在main中声明一个subAB的变量,调用funsame(),理解继承中的二义性,使用函数覆盖的方法解决问题。 2.定义A的子类subA,定义subAA继承A和subA,在main中声明一个subAA的变量,理解重复继承引起的二义性,并使用虚基类的方式解决问题。 3.在A和subA中添加构造函数和析构函数,输出提示;在main中声明一个A的指针apt,赋值为subA的对象 A apt = new subA 运行程序,查看析构函数的调用,使用虚析构函数,观察修改后的结果
时间: 2024-02-11 16:05:26 浏览: 25
1. 代码如下:
```cpp
#include <iostream>
using namespace std;
class A {
public:
int x;
void funsame() {
cout << "This is A's funsame." << endl;
}
};
class B {
public:
int x;
void funsame() {
cout << "This is B's funsame." << endl;
}
};
class xsubAB : public A, public B {
public:
void funsame() {
cout << "This is xsubAB's funsame." << endl;
}
};
int main() {
xsubAB obj;
obj.funsame(); // 输出 This is xsubAB's funsame.
return 0;
}
```
在 `xsubAB` 继承了 `A` 和 `B` 之后,由于 `A` 和 `B` 中都有同名的成员变量和函数,因此在 `xsubAB` 中会产生二义性。为了解决这个问题,我们需要使用函数覆盖,即在 `xsubAB` 中重新实现一个同名函数。
2. 代码如下:
```cpp
#include <iostream>
using namespace std;
class A {
public:
int x;
void funsame() {
cout << "This is A's funsame." << endl;
}
};
class subA : public A {
public:
void funsame() {
cout << "This is subA's funsame." << endl;
}
};
class subAA : virtual public A, virtual public subA {
public:
void funsame() {
cout << "This is subAA's funsame." << endl;
}
};
int main() {
subAA obj;
obj.funsame(); // 输出 This is subAA's funsame.
return 0;
}
```
在 `subAA` 继承了 `A` 和 `subA` 之后,由于 `subA` 中已经继承了 `A`,因此在 `subAA` 中会产生重复继承。为了解决这个问题,我们需要使用虚基类,即在 `A` 和 `subA` 的继承声明中添加 `virtual` 关键字。
3. 代码如下:
```cpp
#include <iostream>
using namespace std;
class A {
public:
int x;
A() {
cout << "A's constructor is called." << endl;
}
virtual ~A() {
cout << "A's destructor is called." << endl;
}
virtual void funsame() {
cout << "This is A's funsame." << endl;
}
};
class subA : public A {
public:
subA() {
cout << "subA's constructor is called." << endl;
}
~subA() {
cout << "subA's destructor is called." << endl;
}
virtual void funsame() {
cout << "This is subA's funsame." << endl;
}
};
int main() {
A* apt = new subA;
apt->funsame(); // 输出 This is subA's funsame.
delete apt;
return 0;
}
```
在 `A` 和 `subA` 中添加了构造函数和析构函数,并且在 `A` 的析构函数和 `subA` 的析构函数中输出了提示信息。在 `main` 函数中,我们声明了一个 `A` 类型的指针 `apt`,并将其赋值为 `subA` 的对象。由于 `A` 的析构函数不是虚函数,因此在删除 `apt` 时只会调用 `A` 的析构函数,而不会调用 `subA` 的析构函数。为了解决这个问题,我们需要使用虚析构函数,即在 `A` 的析构函数前添加 `virtual` 关键字。
相关推荐
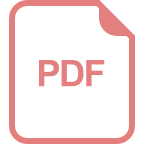
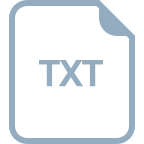
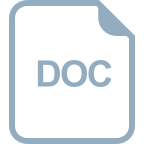
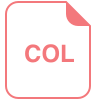
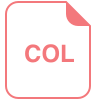
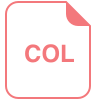
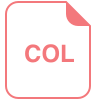
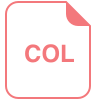









