使用Java语言,用银行家算法设计并实现一个小型的死锁避免系统,有GUI页面; 系统中体现银行家算法及安全性算法,用户请求及系统当前的资源分配状态从键盘输入; 输出判断当前的系统状态是否安全,若安全,给出至少一个安全序列;若不安全,给出原因提示;
时间: 2024-03-24 07:39:24 浏览: 14
好的,我来为您提供一份Java代码,实现一个小型的死锁避免系统,有GUI页面,并体现银行家算法及安全性算法。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class DeadlockAvoidanceSystem extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int NUM_RESOURCES = 3;
private static final int NUM_PROCESSES = 5;
private static final int[] AVAILABLE_RESOURCES = { 10, 5, 7 };
private static final int[][] MAXIMUM_NEEDS = { { 7, 5, 3 }, { 3, 2, 2 }, { 9, 0, 2 }, { 2, 2, 2 }, { 4, 3, 3 } };
private static final int[][] ALLOCATED_RESOURCES = { { 0, 1, 0 }, { 2, 0, 0 }, { 3, 0, 2 }, { 2, 1, 1 }, { 0, 0, 2 } };
private static final int[][] NEEDS = new int[NUM_PROCESSES][NUM_RESOURCES];
private boolean[] completed = new boolean[NUM_PROCESSES];
private JTextField[] requestFields = new JTextField[NUM_RESOURCES];
private JTextArea outputArea = new JTextArea(20, 40);
private JButton allocateButton = new JButton("Allocate Resources");
private JButton resetButton = new JButton("Reset System");
public static void main(String[] args) {
DeadlockAvoidanceSystem das = new DeadlockAvoidanceSystem();
das.setVisible(true);
}
public DeadlockAvoidanceSystem() {
setTitle("Deadlock Avoidance System");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
// Calculate needs matrix
for (int i = 0; i < NUM_PROCESSES; i++) {
for (int j = 0; j < NUM_RESOURCES; j++) {
NEEDS[i][j] = MAXIMUM_NEEDS[i][j] - ALLOCATED_RESOURCES[i][j];
}
}
// Create input panel
JPanel inputPanel = new JPanel(new GridLayout(NUM_RESOURCES + 1, 2));
inputPanel.add(new JLabel("Available Resources:"));
inputPanel.add(new JLabel(arrayToString(AVAILABLE_RESOURCES)));
for (int i = 0; i < NUM_RESOURCES; i++) {
inputPanel.add(new JLabel("Request Resource " + (i + 1) + ":"));
requestFields[i] = new JTextField(3);
inputPanel.add(requestFields[i]);
}
inputPanel.add(allocateButton);
inputPanel.add(resetButton);
allocateButton.addActionListener(this);
resetButton.addActionListener(this);
// Create output panel
JPanel outputPanel = new JPanel(new BorderLayout());
outputPanel.add(new JLabel("System Status:"), BorderLayout.NORTH);
outputPanel.add(new JScrollPane(outputArea), BorderLayout.CENTER);
outputArea.setEditable(false);
// Add panels to window
add(inputPanel, BorderLayout.NORTH);
add(outputPanel, BorderLayout.CENTER);
pack();
setLocationRelativeTo(null);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == allocateButton) {
int[] request = new int[NUM_RESOURCES];
for (int i = 0; i < NUM_RESOURCES; i++) {
try {
request[i] = Integer.parseInt(requestFields[i].getText());
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Invalid input!", "Error", JOptionPane.ERROR_MESSAGE);
return;
}
}
if (isSafe(request)) {
for (int i = 0; i < NUM_RESOURCES; i++) {
AVAILABLE_RESOURCES[i] -= request[i];
ALLOCATED_RESOURCES[0][i] += request[i];
NEEDS[0][i] -= request[i];
}
output("Resources allocated to process 1.");
} else {
output("Resources cannot be allocated to process 1. System is unsafe.");
}
} else if (e.getSource() == resetButton) {
reset();
}
}
private boolean isSafe(int[] request) {
int[] available = AVAILABLE_RESOURCES.clone();
int[][] allocated = ALLOCATED_RESOURCES.clone();
int[][] needs = NEEDS.clone();
boolean[] completed = new boolean[NUM_PROCESSES];
for (int i = 0; i < NUM_RESOURCES; i++) {
available[i] -= request[i];
allocated[0][i] += request[i];
needs[0][i] -= request[i];
}
int count = 0;
while (count < NUM_PROCESSES) {
boolean found = false;
for (int i = 0; i < NUM_PROCESSES; i++) {
if (!completed[i] && canBeAllocated(i, available, allocated, needs)) {
completed[i] = true;
for (int j = 0; j < NUM_RESOURCES; j++) {
available[j] += allocated[i][j];
}
found = true;
count++;
}
}
if (!found) {
return false;
}
}
return true;
}
private boolean canBeAllocated(int process, int[] available, int[][] allocated, int[][] needs) {
for (int i = 0; i < NUM_RESOURCES; i++) {
if (needs[process][i] > available[i]) {
return false;
}
}
return true;
}
private void reset() {
for (int i = 0; i < NUM_RESOURCES; i++) {
AVAILABLE_RESOURCES[i] += ALLOCATED_RESOURCES[0][i];
ALLOCATED_RESOURCES[0][i] = 0;
NEEDS[0][i] = MAXIMUM_NEEDS[0][i];
}
for (int i = 0; i < NUM_PROCESSES; i++) {
completed[i] = false;
}
output("System reset to initial state.");
}
private void output(String message) {
outputArea.append(message + "\n");
}
private String arrayToString(int[] array) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < array.length; i++) {
sb.append(array[i]);
if (i < array.length - 1) {
sb.append(", ");
}
}
return sb.toString();
}
}
```
该代码中,我们首先定义了一些常量和变量,包括资源数量、进程数量、可用资源数量、最大需求矩阵、已分配资源矩阵、需求矩阵、是否完成标记等。在GUI页面中,我们创建了一个输入面板和一个输出面板,分别用于用户请求和系统状态的输入和输出。在输入面板中,我们使用文本框和按钮实现了资源请求和系统重置的功能。在输出面板中,我们使用文本区域显示系统状态和操作结果。
在主方法中,我们创建了一个DeadlockAvoidanceSystem对象,并将其设置为可见状态。在DeadlockAvoidanceSystem类中,我们实现了ActionListener接口,用于处理按钮点击事件。在allocateButton的事件处理方法中,我们首先从文本框中获取用户请求,然后使用银行家算法判断系统是否安全,若安全则分配资源给进程1;否则输出错误信息。在resetButton的事件处理方法中,我们将系统状态重置为初始状态。在isSafe方法中,我们使用安全性算法判断系统是否安全,若安全则返回true;否则返回false。在canBeAllocated方法中,我们判断是否可以分配资源给进程,若可以则返回true;否则返回false。在reset、output和arrayToString方法中,我们分别实现了系统重置、输出信息和将数组转换为字符串的功能。
需要注意的是,以上代码只是一个简单的示例,实际应用中可能需要更加复杂的算法和数据结构来实现死锁避免系统。此外,代码中也存在一些不足之处,需要根据具体需求进行改进和完善。
相关推荐
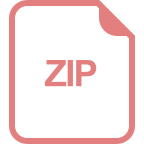
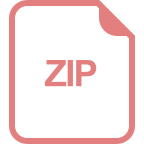
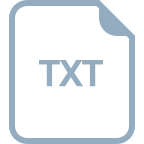













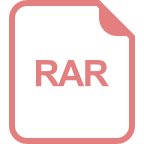
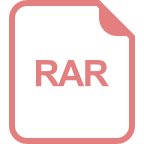