写一个QT程序:点击按纽开始计时,2分钟得到一个次数,20分钟后停止
时间: 2024-01-22 08:17:47 浏览: 24
以下是一个简单的QT程序,可以实现点击按钮后开始计时,计时2分钟后输出一个随机次数,20分钟后自动停止计时。
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QWidget>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QLabel>
#include <QtCore/QTimer>
#include <QtCore/QTime>
#include <QtCore/QRandomGenerator>
class TimeCounter : public QWidget {
public:
TimeCounter(QWidget *parent = nullptr) : QWidget(parent) {
setFixedSize(400, 200);
setWindowTitle("Time Counter");
counterLabel = new QLabel(this);
counterLabel->setGeometry(50, 50, 300, 50);
counterLabel->setText("Click the button to start counting.");
startButton = new QPushButton(this);
startButton->setGeometry(150, 120, 100, 50);
startButton->setText("Start");
connect(startButton, &QPushButton::clicked, this, &TimeCounter::startCounting);
timer = new QTimer(this);
connect(timer, &QTimer::timeout, this, &TimeCounter::updateCount);
}
private:
QLabel *counterLabel;
QPushButton *startButton;
QTimer *timer;
int count = 0;
void startCounting() {
count = 0;
counterLabel->setText("Counting...");
timer->start(1000 * 60 * 2); // 2 minutes
}
void updateCount() {
if (count == 0) {
counterLabel->setText(QString("Random count: %1").arg(QRandomGenerator::global()->bounded(100)));
}
count++;
if (count == 20) {
counterLabel->setText("Counting stopped.");
timer->stop();
}
}
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
TimeCounter timeCounter;
timeCounter.show();
return app.exec();
}
```
在这个程序中,我们创建了一个 `TimeCounter` 类,继承自 `QWidget`。它包含一个标签用于显示计数器的状态和结果,一个按钮用于开始计时,一个计时器用于计时并更新计数器。
当用户点击按钮时,我们启动计时器,并在计时器到达指定时间时更新计数器的状态。在计时器到达第一次更新的时间时,我们使用 `QRandomGenerator` 生成一个随机数作为计数器的值。
当计时器到达最大时间时,我们停止计时器并更新计数器的状态。
相关推荐
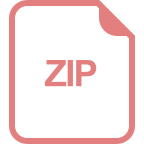
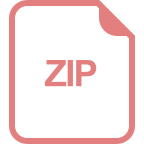














