c语言链表实现集合的交,并,补,差运算,并且可以控制台交互
时间: 2024-04-03 15:36:34 浏览: 16
好的,下面是使用链表实现集合的交、并、补、差运算,并且可以在控制台进行交互的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int data;
struct node* next;
} Node;
// 创建一个新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 插入一个数值到链表中
void insert(Node** headRef, int data) {
Node* newNode = createNode(data);
Node* last = *headRef;
if (*headRef == NULL) {
*headRef = newNode;
return;
}
while (last->next != NULL) {
last = last->next;
}
last->next = newNode;
}
// 遍历链表
void traverse(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 集合的并运算
Node* unionSet(Node* a, Node* b) {
Node* result = NULL;
Node** lastPtrRef = &result;
while (a != NULL && b != NULL) {
if (a->data < b->data) {
*lastPtrRef = a;
a = a->next;
}
else if (a->data > b->data) {
*lastPtrRef = b;
b = b->next;
}
else {
*lastPtrRef = a;
a = a->next;
b = b->next;
}
lastPtrRef = &((*lastPtrRef)->next);
}
if (a != NULL) {
*lastPtrRef = a;
}
else {
*lastPtrRef = b;
}
return result;
}
// 集合的交运算
Node* intersectionSet(Node* a, Node* b) {
Node* result = NULL;
Node** lastPtrRef = &result;
while (a != NULL && b != NULL) {
if (a->data < b->data) {
a = a->next;
}
else if (a->data > b->data) {
b = b->next;
}
else {
*lastPtrRef = createNode(a->data);
a = a->next;
b = b->next;
lastPtrRef = &((*lastPtrRef)->next);
}
}
return result;
}
// 集合的差运算
Node* differenceSet(Node* a, Node* b) {
Node* result = NULL;
Node** lastPtrRef = &result;
while (a != NULL && b != NULL) {
if (a->data < b->data) {
*lastPtrRef = createNode(a->data);
a = a->next;
lastPtrRef = &((*lastPtrRef)->next);
}
else if (a->data > b->data) {
b = b->next;
}
else {
a = a->next;
b = b->next;
}
}
while (a != NULL) {
*lastPtrRef = createNode(a->data);
a = a->next;
lastPtrRef = &((*lastPtrRef)->next);
}
return result;
}
// 集合的补运算
Node* complementSet(Node* a, Node* b) {
Node* result = NULL;
Node** lastPtrRef = &result;
while (a != NULL && b != NULL) {
if (a->data < b->data) {
*lastPtrRef = createNode(a->data);
a = a->next;
lastPtrRef = &((*lastPtrRef)->next);
}
else if (a->data > b->data) {
b = b->next;
}
else {
a = a->next;
b = b->next;
}
}
while (a != NULL) {
*lastPtrRef = createNode(a->data);
a = a->next;
lastPtrRef = &((*lastPtrRef)->next);
}
return result;
}
// 释放链表内存
void freeList(Node* head) {
Node* current = head;
Node* next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
}
int main() {
int choice, data;
Node *a = NULL, *b = NULL, *result = NULL;
while (1) {
printf("1. Insert into set A\n");
printf("2. Insert into set B\n");
printf("3. Union of sets A and B\n");
printf("4. Intersection of sets A and B\n");
printf("5. Difference of sets A and B\n");
printf("6. Complement of sets A and B\n");
printf("7. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter data to insert into set A: ");
scanf("%d", &data);
insert(&a, data);
printf("Set A: ");
traverse(a);
break;
case 2:
printf("Enter data to insert into set B: ");
scanf("%d", &data);
insert(&b, data);
printf("Set B: ");
traverse(b);
break;
case 3:
result = unionSet(a, b);
printf("Union of sets A and B: ");
traverse(result);
freeList(result);
break;
case 4:
result = intersectionSet(a, b);
printf("Intersection of sets A and B: ");
traverse(result);
freeList(result);
break;
case 5:
result = differenceSet(a, b);
printf("Difference of sets A and B: ");
traverse(result);
freeList(result);
break;
case 6:
result = complementSet(a, b);
printf("Complement of sets A and B: ");
traverse(result);
freeList(result);
break;
case 7:
freeList(a);
freeList(b);
exit(0);
default:
printf("Invalid choice!\n");
break;
}
}
return 0;
}
```
运行程序后,可以选择需要进行的操作,插入数据到集合A或集合B,或进行集合的并、交、差、补运算。
相关推荐
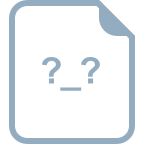














