vue使用konva.js实现多边形拖拽
时间: 2023-09-02 22:04:07 浏览: 97
Vue可以使用Konva.js库来实现多边形的拖拽效果。以下是一个简单的实现示例:
1. 首先,我们需要在Vue项目中引入Konva.js库。可以通过npm安装或直接引入CDN链接。
2. 在Vue组件中,我们可以通过使用Konva的Stage和Layer组件来创建画布和图层。
3. 在data中定义多边形的坐标和其他相关属性。例如,我们可以定义一个数组polygons,每个元素包含多边形的id、坐标和颜色信息。
4. 在mounted钩子函数中,初始化Konva实例并为每个多边形创建Konva的Shape对象,并将其添加到Layer中。
5. 实现拖拽功能,可以使用Konva的dragBoundFunc属性来限制拖拽的范围。我们可以通过添加事件处理程序来监听拖拽事件,并更新多边形的坐标。
6. 将Layer添加到Stage中,并在template中将Stage渲染到页面上。
下面是一个简单示例组件的代码:
```
<template>
<div>
<div ref="stageContainer"></div>
</div>
</template>
<script>
import Konva from 'konva'
export default {
data() {
return {
polygons: [
{ id: 1, points: [100, 100, 200, 100, 150, 200], color: 'blue' },
{ id: 2, points: [300, 100, 400, 100, 350, 200], color: 'red' },
],
};
},
mounted() {
const stage = new Konva.Stage({
container: this.$refs.stageContainer,
width: 500,
height: 300
});
const layer = new Konva.Layer();
stage.add(layer);
this.polygons.forEach(polygon => {
const shape = new Konva.Line({
points: polygon.points,
fill: polygon.color,
draggable: true,
dragBoundFunc: function (pos) {
const stageWidth = stage.width();
const stageHeight = stage.height();
const shapeWidth = this.width();
const shapeHeight = this.height();
const x = pos.x < 0 ? 0 : pos.x;
const y = pos.y < 0 ? 0 : pos.y;
return {
x: x > stageWidth - shapeWidth ? stageWidth - shapeWidth : x,
y: y > stageHeight - shapeHeight ? stageHeight - shapeHeight : y
};
}
});
layer.add(shape);
shape.on('dragmove', () => {
polygon.points = shape.points();
});
});
},
};
</script>
```
以上示例演示了如何使用Vue和Konva.js库实现多边形的拖拽效果。请注意,这只是一个简单的示例,并且可以根据具体需求进行适当的修改和扩展。
相关推荐
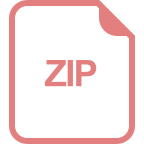
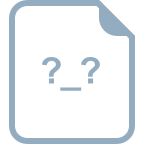
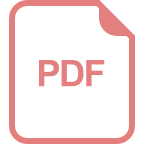














