Explain, in your own words, what an overloaded method is in the Java Language, give an example of where you would use an overloaded method, and explain why.
时间: 2024-03-14 16:48:03 浏览: 100
An overloaded method is a method in Java that has the same name as another method in the same class, but has different parameters. The parameters can differ in number, type, or order. When a method is called, the Java compiler will determine which version of the method to use based on the arguments passed in.
For example, consider a class that has a method called "add" that adds two integers together. We could overload this method by creating another method called "add" that takes three integers and adds them together. Here's an example:
```
public class Calculator {
public int add(int x, int y) {
return x + y;
}
public int add(int x, int y, int z) {
return x + y + z;
}
}
```
We could then use these methods like this:
```
Calculator calc = new Calculator();
int sum1 = calc.add(2, 3); // calls the first "add" method
int sum2 = calc.add(2, 3, 4); // calls the second "add" method
```
We would use overloaded methods when we want the same functionality with varying inputs. In the above example, we want to be able to add two or three integers together, so we overload the "add" method to accommodate both cases. This makes our code more flexible and easier to use.
阅读全文
相关推荐




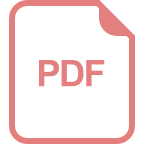









