如何通过python 不断的查询elasticsearch中符合某个条件的数据,并且查询的结果不重复,并且一旦有新数据插入也可以即时的检测到,给出详细的代码和解释
时间: 2024-06-10 21:07:16 浏览: 144
可以使用Elasticsearch提供的scroll api进行持续的查询,并使用Python的set数据类型来去重。可以使用Elasticsearch的watcher功能来实现对于新数据的及时检测。
以下是示例代码:
```python
from elasticsearch import Elasticsearch
import time
es = Elasticsearch("localhost:9200")
query = {
"query": {
"match": {
"your_field": "your_value"
}
}
}
scroll_timeout = "1m"
# 设置scroll参数
search_params = {
"scroll": scroll_timeout,
"size": 1000,
"index": "your_index",
"body": query,
"sort": ["_doc"]
}
# 初始化scroll
response = es.search(**search_params)
scroll_id = response.get("_scroll_id")
# 初始化结果集
results_set = set()
while True:
# 遍历scroll结果
for hit in response["hits"]["hits"]:
# 将结果加入结果集
results_set.add(hit["_id"])
# 如果结果集发生变化,进行处理
if len(results_set) > 0:
print("New results: ", results_set)
# 清空结果集
results_set.clear()
# 检查是否有新数据插入
time.sleep(1)
new_hits = es.scroll(scroll_id=scroll_id, scroll=scroll_timeout)["hits"]["hits"]
if len(new_hits) == 0:
# 如果无新数据,则退出循环
break
# 更新scroll_id
scroll_id = response["_scroll_id"]
```
以上代码首先进行一次查询并获取scroll_id,然后在无限循环中使用scroll_id不断进行查询,直至taple scrolled api返回的结果为空。每次查询到新数据后,将其加入结果集中并进行处理,最后清空结果集以待处理下一批数据。如果需要实时检测新数据,可以将上述代码嵌入到一个无限循环中,定时运行即可。
阅读全文
相关推荐
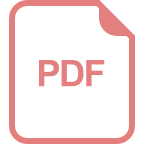
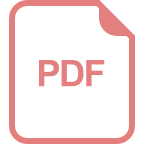
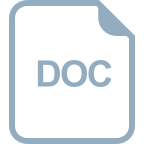
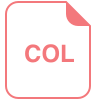
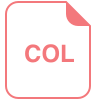
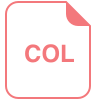
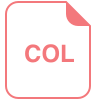
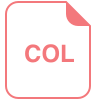
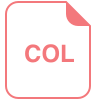
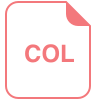
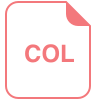
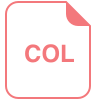
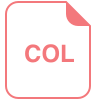
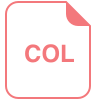
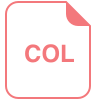
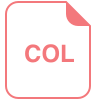
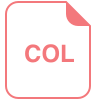
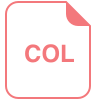