c语言单链表从小到大冒泡排序
时间: 2024-12-09 22:11:29 浏览: 40
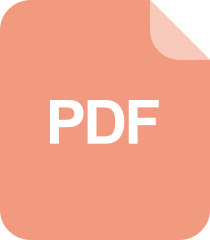
C语言排序算法大全
在C语言中,单链表的冒泡排序是一种常见的排序算法。冒泡排序通过重复地遍历要排序的数列,一次比较两个元素,如果它们的顺序错误就交换过来。由于单链表的特性,我们需要在遍历链表时进行节点交换。以下是一个简单的示例代码,展示了如何对单链表进行从小到大的冒泡排序。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("内存分配失败\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表末尾
void append(Node** head_ref, int new_data) {
Node* newNode = createNode(new_data);
if (*head_ref == NULL) {
*head_ref = newNode;
return;
}
Node* last = *head_ref;
while (last->next != NULL)
last = last->next;
last->next = newNode;
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
// 冒泡排序
void bubbleSort(Node* head) {
int swapped;
Node* ptr1;
Node* lptr = NULL;
if (head == NULL)
return;
do {
swapped = 0;
ptr1 = head;
while (ptr1->next != lptr) {
if (ptr1->data > ptr1->next->data) {
// 交换数据
int temp = ptr1->data;
ptr1->data = ptr1->next->data;
ptr1->next->data = temp;
swapped = 1;
}
ptr1 = ptr1->next;
}
lptr = ptr1;
} while (swapped);
}
int main() {
Node* head = NULL;
// 创建链表
append(&head, 5);
append(&head, 1);
append(&head, 4);
append(&head, 2);
append(&head, 3);
printf("原始链表: ");
printList(head);
// 冒泡排序
bubbleSort(head);
printf("排序后链表: ");
printList(head);
return 0;
}
```
这段代码首先定义了一个单链表节点结构,并实现了创建新节点、插入节点到链表末尾以及打印链表的功能。然后,通过冒泡排序算法对链表进行排序。
阅读全文
相关推荐
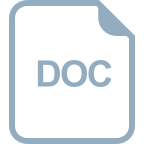
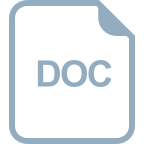
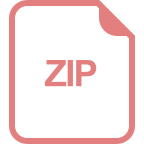
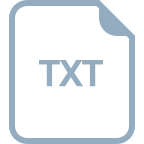
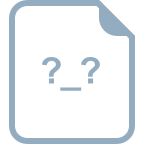
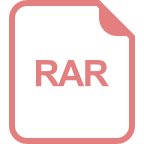
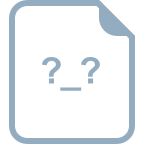
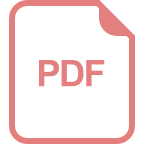
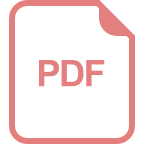
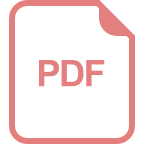