c++设计算法判断一个字符序列是否为回文序列,所谓回文序列就是正读与反读都相同的字符序列,例如:abdba是回文序列(要求采用链栈来实现)。
时间: 2023-05-20 21:02:32 浏览: 55
可以使用链栈来实现判断一个字符序列是否为回文序列的算法,具体步骤如下:
1. 将字符序列依次入栈,同时记录字符序列的长度。
2. 从栈顶开始依次出栈,将出栈的字符依次与原字符序列的每个字符比较,如果有不相同的字符,则说明不是回文序列,直接返回 false。
3. 如果所有字符都相同,则说明是回文序列,返回 true。
以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_SIZE 100
typedef struct Node {
char data;
struct Node *next;
} Node, *LinkStack;
LinkStack InitStack() {
LinkStack S = (LinkStack)malloc(sizeof(Node));
S->next = NULL;
return S;
}
bool IsEmpty(LinkStack S) {
return S->next == NULL;
}
void Push(LinkStack S, char x) {
Node *p = (Node*)malloc(sizeof(Node));
p->data = x;
p->next = S->next;
S->next = p;
}
char Pop(LinkStack S) {
if (IsEmpty(S)) {
printf("Stack is empty!\n");
exit(1);
}
Node *p = S->next;
char x = p->data;
S->next = p->next;
free(p);
return x;
}
bool IsPalindrome(char *str) {
LinkStack S = InitStack();
int len = 0;
while (str[len] != '\0') {
Push(S, str[len]);
len++;
}
for (int i = 0; i < len; i++) {
if (Pop(S) != str[i]) {
return false;
}
}
return true;
}
int main() {
char str[MAX_SIZE];
printf("Please input a string: ");
scanf("%s", str);
if (IsPalindrome(str)) {
printf("The string is a palindrome.\n");
} else {
printf("The string is not a palindrome.\n");
}
return 0;
}
```
输入示例:
```
Please input a string: abdba
```
输出示例:
```
The string is a palindrome.
```
相关推荐
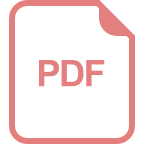
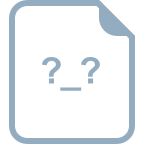
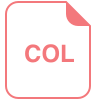
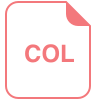







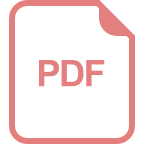
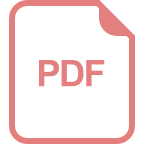
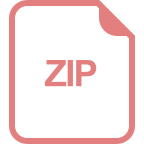