在开发C语言的商品信息管理系统时,如何设计一个包含输入输出、查找、修改和删除功能的模块?要求不使用链表,而是采用文件作为数据存储方式,请详细说明实现步骤和技术细节,每个都要详细的注释
时间: 2024-12-17 16:28:08 浏览: 1
在C语言中,设计一个基于文件的商品信息管理系统,我们将创建四个基本模块:输入(录入商品信息)、输出(显示商品列表)、查找(检索特定商品)和修改/删除(更新或移除商品)。这里我们假设商品信息由ID、名称和价格组成,存储在一个逗号分隔的文本文件中。
### 第一步:定义结构体和宏
首先,定义一个商品结构体:
```c
// 定义商品结构体
typedef struct {
int id;
char name[50];
double price;
} Product;
```
然后创建用于读写文件的操作宏:
```c
// 文件操作宏
#define FILE_NAME "products.txt"
#define OPEN_FILE(flag) fopen(FILE_NAME flag, "a+")
#define READ_LINE(file) fgets(line, sizeof(line), file)
#define WRITE_LINE(file, product) fprintf(file, "%d,%s,%lf\n", product.id, product.name, product.price)
#define READ_PRODUCT(file, product) sscanf(line, "%d,%[^,],%lf", &product.id, product.name, &product.price)
#define DELETE_LINE(file, id) fseek(file, -strlen(strcat(id, ",")), SEEK_END); truncate(file);
#define MODIFY_LINE(file, id, new_product) { \
char *line_to_modify = malloc(strlen(line) + 1); \
fgets(line_to_modify, sizeof(line_toModify), file); \
sscanf(line_to_modify, "%d,%[^,],%lf", &product.id, product.name, &product.price); \
product.price = new_product.price; \
sprintf(line_toModify, "%d,%s,%lf", product.id, product.name, product.price); \
fseek(file, -strlen(line_to_modify), SEEK_END); \
free(line_to_modify); \
fwrite(line_toModify, 1, strlen(line_toModify), file); \
}
```
### 第二步:模块实现
1. **输入模块**:
```c
void input(Product* products) {
FILE* file = OPEN_FILE("");
if (!file) {
printf("无法打开文件.\n");
return;
}
int choice, product_id;
while (1) {
printf("请选择操作:1. 新增商品 2. 查看所有商品 3. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入商品ID, 名称, 价格:\n");
scanf("%d,%[^,],%lf", &product_id, product.name, &product.price);
WRITE_LINE(file, products[product_count++]);
break;
// 其他选项...
}
}
fclose(file);
}
```
2. **输出模块**:
```c
void output() {
FILE* file = OPEN_FILE("");
if (!file) {
printf("无法打开文件.\n");
return;
}
int product_count = 0;
char line[100];
while (READ_LINE(file)) {
++product_count;
READ_PRODUCT(file, products[product_count-1]);
printf("%d. ID: %d, Name: %s, Price: %.2lf\n", product_count, products[product_count-1].id, products[product_count-1].name, products[product_count-1].price);
}
fclose(file);
}
```
3. **查找模块**:
```c
Product searchProduct(int id) {
FILE* file = OPEN_FILE("");
Product found_product;
if (READ_LINE(file)) {
int index = 0;
while (index < product_count && products[index].id != id) {
++index;
READ_PRODUCT(file, products[index]);
}
if (index < product_count) {
return products[index];
} else {
printf("未找到ID为%d的产品.\n", id);
}
} else {
printf("文件为空.\n");
}
fclose(file);
return found_product;
}
```
4. **修改/删除模块**:
```c
void modifyOrDeleteProduct(int id, double new_price = 0) {
FILE* file = OPEN_FILE("");
if (!file) {
printf("无法打开文件.\n");
return;
}
for (int i = 0; i < product_count; ++i) {
if (products[i].id == id) {
if (new_price > 0) {
MODIFY_LINE(file, to_string(i), new_price);
} else {
DELETE_LINE(file, to_string(i));
}
break;
}
}
fclose(file);
}
```
请注意,以上代码仅做示例,实际项目中需增加错误处理和边界条件检查。另外,为了提高代码的可维护性和安全性,建议将数据结构封装成单独的模块,并考虑使用预编译头文件管理文件路径和操作。
阅读全文
相关推荐
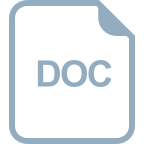
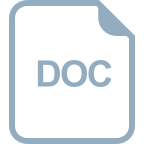
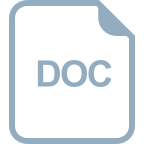
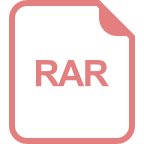














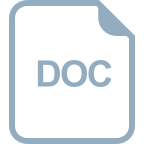