请通过C语言具体实现以上步骤
时间: 2024-12-15 22:22:22 浏览: 1
在C语言中实现上述步骤涉及到多个库和技术,这里是一个简化的示例,仅展示关键的部分,因为你提到的DHCP获取IP和向电脑发送的具体细节依赖于操作系统和底层库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libusb.h>
// 假设我们有一个函数handle_usb_device,负责与USB设备交互
void handle_usb_device(libusb_device* device) {
// ...在这里添加与设备交互的具体代码...
// 假设设备支持发送数据
if (send_data_to_device(device)) {
printf("Sent IP address to device.\n");
} else {
perror("Failed to send data to device.");
}
}
// 假设存在一个函数dhcp_get_ip,从系统中获取IP
char* dhcp_get_ip() {
// 使用getifaddrs()等系统调用来获取IP
struct ifaddrs *ifa;
char ip[INET_ADDRSTRLEN];
// ...在此处实现DHCP获取IP的过程...
return ip;
}
int main(int argc, char** argv) {
libusb_context* ctx = libusb_init(NULL);
if (ctx == NULL) {
perror("Error initializing USB context");
exit(EXIT_FAILURE);
}
// 搜索并打开USB设备
libusb_device* dev = find_and_open_usb_device(ctx, "VendorID", "ProductID"); // 替换为实际的 Vendor ID 和 Product ID
if (dev == NULL) {
perror("Could not find USB device");
libusb_exit(ctx);
exit(EXIT_FAILURE);
}
// 获取IP
char* ip = dhcp_get_ip();
if (ip == NULL) {
perror("Failed to get IP from DHCP");
libusb_close(dev);
libusb_exit(ctx);
exit(EXIT_FAILURE);
}
// 执行设备处理
handle_usb_device(dev);
// 清理资源
free(ip);
libusb_close(dev);
libusb_exit(ctx);
return 0;
}
```
请注意,这个例子非常简化,实际实现中可能需要额外处理错误、设备枚举以及可能涉及的USB数据传输格式。此外,DHCP获取IP通常是操作系统层面的工作,而这里只是一个理论上的框架。
阅读全文
相关推荐











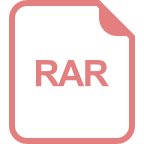

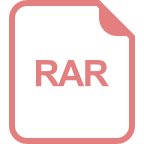




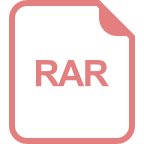