python安装lda
时间: 2023-11-14 14:07:59 浏览: 75
根据提供的引用,我们可以使用以下命令来安装lda库:
```python
pip install pyLDAvis
```
如果您的Python环境中有多个版本,则需要使用以下命令来确保在正确的Python版本中安装lda库:
```python
python -m pip install pyLDAvis
```
请注意,您需要在命令提示符或终端中运行此命令,并且需要已经安装了pip。如果您还没有安装pip,请参考官方文档进行安装。
相关问题
python 实习LDA
好的,关于 Python 实现 LDA,我可以给你一些简单的介绍。
LDA(Latent Dirichlet Allocation)是一种主题模型,用于发现文本集合中的隐藏主题。在 Python 中,有很多第三方库可以用来实现 LDA,比如 gensim 和 sklearn 等。
下面是一个使用 gensim 库实现 LDA 的简单示例代码:
```python
from gensim import corpora, models
# 构建词典
dictionary = corpora.Dictionary(texts)
# 将文本转换为词袋表示
corpus = [dictionary.doc2bow(text) for text in texts]
# 训练 LDA 模型
lda_model = models.ldamodel.LdaModel(corpus=corpus, id2word=dictionary, num_topics=10)
# 输出每个主题的关键词
for topic in lda_model.print_topics(num_topics=10, num_words=10):
print(topic)
```
其中,`texts` 是一个包含多个文本的列表,每个文本又是一个包含多个词语的列表。`num_topics` 参数指定了要发现的主题数。
Python实现LDA
LDA(Latent Dirichlet Allocation)是一种常用的主题模型,用于从文本集合中发现隐藏的主题结构。下面是使用Python实现LDA的一种方法:
首先,你需要安装gensim库,它提供了一个简单的接口来实现LDA。可以通过以下命令安装:
```
pip install gensim
```
接下来,导入所需的库:
```python
import gensim
from gensim import corpora
from gensim.models import LdaModel
```
然后,准备数据。将文本集合转换为词袋表示,这可以通过分词和创建词袋模型来完成。以下是一个简单的示例:
```python
documents = ["I like to play football",
"I enjoy playing soccer",
"Football is my favorite sport",
"I love watching basketball games"]
# 分词
tokenized_documents = [document.lower().split() for document in documents]
# 创建词袋模型
dictionary = corpora.Dictionary(tokenized_documents)
# 将文本转换为词袋表示
corpus = [dictionary.doc2bow(document) for document in tokenized_documents]
```
现在,可以使用LdaModel类来训练主题模型并执行推断。以下是一个简单的示例:
```python
# 训练LDA模型
lda_model = LdaModel(corpus=corpus,
id2word=dictionary,
num_topics=2,
passes=10)
# 打印每个主题的关键词
topics = lda_model.print_topics()
for topic in topics:
print(topic)
```
以上代码将训练一个包含2个主题的LDA模型,并打印每个主题的关键词。
这只是LDA的一个简单实现示例,gensim库还提供了更多高级功能和参数选项,你可以根据自己的需求进行调整和扩展。
相关推荐
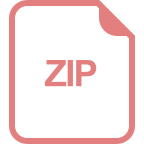












