在C语言中如果要用get()怎么编写代码
时间: 2024-09-15 18:15:33 浏览: 37
在C语言中,`get()` 函数通常不是标准库的一部分,因为它的语法和作用不太明确。如果你想获取用户输入,应该使用标准库函数如`scanf()`、`fgets()`或者`getline()`。这里给出一个简单的例子,展示如何使用`fgets()`函数从标准输入(通常是键盘)获取一行文本:
```c
#include <stdio.h>
#include <string.h> // 包含字符串处理函数
#define MAX_LINE_LENGTH 80 // 设置最大行长度,可以根据需求调整
int main() {
char input[MAX_LINE_LENGTH]; // 定义字符数组存储输入
printf("请输入一些文本: ");
fgets(input, MAX_LINE_LENGTH, stdin); // 从stdin读取到input数组,直到换行符
// 移除字符串末尾的换行符(如果有的话)
size_t len = strlen(input);
if (len > 0 && input[len - 1] == '\n') {
input[len - 1] = '\0'; // 将最后一个字符设为'\0'
}
printf("你输入的内容是: %s\n", input);
return 0;
}
```
如果你是指网络请求中的 `get` 函数,那可能是某个HTTP客户端库提供的API,如 cURL。但在标准C环境中并没有这样的函数。
相关问题
用C语言编写上述代码
当然,以下是使用C语言编写的三种查找算法代码:
(1) 无序链表查找:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* linearSearchUnsorted(Node* head, int z) {
Node* current = head;
while (current != NULL && current->data != z) {
current = current->next;
}
return current;
}
```
(2) 递增有序链表查找(二分查找):
```c
Node* getMiddle(Node* low, Node* high) {
if (low == high) return low;
Node* mid = low->next;
for (int i = 1; i < (high - low).size / 2; i++) {
mid = mid->next;
}
return mid;
}
Node* binarySearchSorted(Node* head, int z) {
if (head == NULL || head->data > z) return NULL;
Node* low = head;
Node* high = head->next;
while (low != high) {
Node* mid = getMiddle(low, high);
if (mid->data < z) low = mid->next;
else high = mid;
}
return high;
}
```
(3) 递减有序链表查找(二分查找):
```c
Node* binarySearchDecreasingSorted(Node* head, int z) {
if (head == NULL || head->data <= z) return NULL;
Node* low = head;
Node* high = head->prev; // 初始化高指针为前一个节点
while (low != high) {
Node* mid = getMiddle(low, high);
if (mid->data > z) low = mid->prev;
else high = mid;
}
return low;
}
```
注意这里的链表操作可能需要根据实际的数据结构库进行调整。例如,`getMiddle`函数假设链表节点是一个数组,但在实际中可能需要对链表进行迭代计算。
用c语言编写代码 利用指针,设计两数求一维数组中的平均值
好的,让我来回答你的问题。这里提供一种用C语言编写代码,利用指针,设计两数求一维数组中的平均值的方法:
```c
#include <stdio.h>
// 函数声明
double get_average(int *arr, int size);
int main()
{
int num1 = 5, num2 = 10;
int num_array[] = {2, 4, 6, 8, 10};
int *arr_ptr = num_array; // 定义一个指向数组的指针
double average;
// 调用函数,求num1, num2和数组中所有数字的平均值
average = get_average(&num1, 2);
printf("Average of num1 and num2: %f\n", average);
average = get_average(num_array, 5);
printf("Average of the array: %f\n", average);
return 0;
}
double get_average(int *arr, int size)
{
int i, sum = 0;
double average;
for (i = 0; i < size; ++i)
{
sum += *(arr + i); // 通过指针访问数组元素
}
average = (double)sum / size;
return average;
}
```
以上程序中,我们传递了两个参数给 `get_average()` 函数:一个指向数组的指针和数组元素的个数。在函数中,我们通过指针访问数组元素,并计算出平均值并返回。在 `main()` 函数中,我们展示了如何传递两个变量和一个数组,以获取它们的平均值。
阅读全文
相关推荐
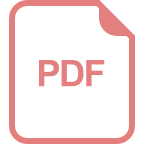
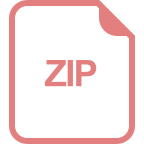
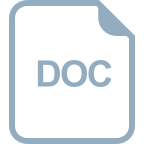
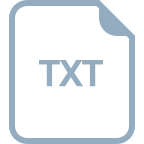
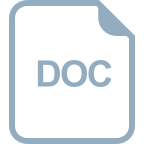
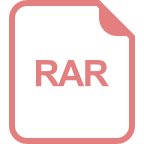
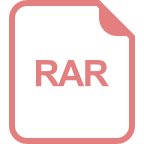
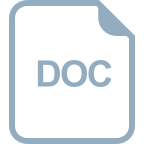
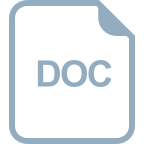
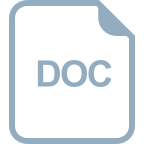
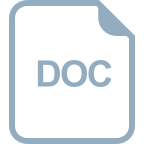
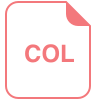



