带有登录系统的仓库管理系统代码c语言
时间: 2023-12-23 15:05:05 浏览: 124
当然,下面是一个简单的仓库管理系统的代码示例,包含了登录系统的功能。这个代码使用了C语言来实现,你可以根据自己的需求进行修改和扩展。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 仓库物品结构体
typedef struct {
char name[50];
int quantity;
} Item;
// 用户结构体
typedef struct {
char username[50];
char password[50];
} User;
// 全局变量
User currentUser; // 当前登录用户
Item items[100]; // 仓库物品数组
int itemCount = 0; // 当前仓库物品数量
// 登录函数
void login() {
printf("请输入用户名: ");
scanf("%s", currentUser.username);
printf("请输入密码: ");
scanf("%s", currentUser.password);
printf("登录成功!\n");
}
// 添加物品函数
void addItem() {
printf("请输入物品名称: ");
scanf("%s", items[itemCount].name);
printf("请输入物品数量: ");
scanf("%d", &items[itemCount].quantity);
itemCount++;
printf("物品添加成功!\n");
}
// 显示所有物品函数
void showItems() {
printf("仓库物品列表:\n");
for (int i = 0; i < itemCount; i++) {
printf("物品名称: %s, 数量: %d\n", items[i].name, items[i].quantity);
}
}
int main() {
int choice;
while (1) {
printf("欢迎使用仓库管理系统!\n");
printf("1. 登录\n");
printf("2. 添加物品\n");
printf("3. 显示所有物品\n");
printf("4. 退出\n");
printf("请选择操作: ");
scanf("%d", &choice);
switch (choice) {
case 1:
login();
break;
case 2:
if (strlen(currentUser.username) == 0) {
printf("请先登录!\n");
} else {
addItem();
}
break;
case 3:
if (strlen(currentUser.username) == 0) {
printf("请先登录!\n");
} else {
showItems();
}
break;
case 4:
printf("谢谢使用!\n");
exit(0);
default:
printf("无效的选项!\n");
}
printf("\n");
}
return 0;
}
```
这个代码实现了一个简单的仓库管理系统,包含了登录功能、添加物品和显示物品列表的功能。你可以根据自己的需求进行修改和扩展。注意,这个代码只是一个基本的示例,没有进行用户验证和数据持久化处理,如果需要更复杂的功能,可以在此基础上进行扩展。
阅读全文
相关推荐
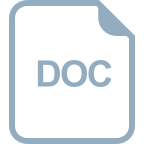
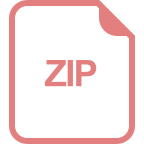
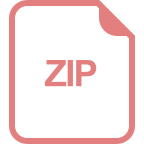















