C++实现ATM系统,并运用链表的增删改查
时间: 2024-03-07 07:50:54 浏览: 77
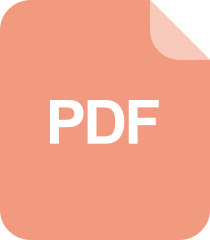
关于双向链表的增删改查和排序的C++实现
好的,我可以为您提供一个简单的C++实现的ATM系统,并使用链表来实现增删改查功能。
首先,我们需要定义一个银行账户类(Account),包含账户号码(account_number),账户余额(balance)和账户持有人姓名(owner_name)等属性。定义如下:
```cpp
class Account {
public:
Account(int account_number, string owner_name, double balance = 0.0) :
account_number(account_number), owner_name(owner_name), balance(balance) {}
int getAccountNumber() const { return account_number; }
double getBalance() const { return balance; }
string getOwnerName() const { return owner_name; }
void deposit(double amount) { balance += amount; }
bool withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
return true;
}
return false;
}
private:
int account_number;
string owner_name;
double balance;
};
```
接下来,我们需要定义一个链表节点类(Node),包含指向账户对象的指针(account)和指向下一个节点的指针(next)属性。定义如下:
```cpp
class Node {
public:
Node(Account* account) : account(account), next(nullptr) {}
Account* getAccount() const { return account; }
Node* getNext() const { return next; }
void setNext(Node* node) { next = node; }
private:
Account* account;
Node* next;
};
```
最后,我们定义一个链表类(LinkedList),包含头节点属性(head)和相应的增删改查方法。定义如下:
```cpp
class LinkedList {
public:
LinkedList() : head(nullptr) {}
void add(Account* account) {
Node* node = new Node(account);
node->setNext(head);
head = node;
}
bool remove(int account_number) {
Node* prev = nullptr;
Node* curr = head;
while (curr != nullptr) {
if (curr->getAccount()->getAccountNumber() == account_number) {
if (prev == nullptr) {
head = curr->getNext();
} else {
prev->setNext(curr->getNext());
}
delete curr;
return true;
}
prev = curr;
curr = curr->getNext();
}
return false;
}
Account* get(int account_number) const {
Node* curr = head;
while (curr != nullptr) {
if (curr->getAccount()->getAccountNumber() == account_number) {
return curr->getAccount();
}
curr = curr->getNext();
}
return nullptr;
}
void update(Account* account) {
Account* acc = get(account->getAccountNumber());
if (acc != nullptr) {
acc->deposit(account->getBalance());
}
}
private:
Node* head;
};
```
现在,我们可以在主函数中创建一个链表对象,并对其进行增删改查操作。示例代码如下:
```cpp
int main() {
LinkedList list;
Account* account1 = new Account(1001, "Alice", 1000);
Account* account2 = new Account(1002, "Bob", 2000);
Account* account3 = new Account(1003, "Charlie", 3000);
list.add(account1);
list.add(account2);
list.add(account3);
// 查询账户信息
Account* acc = list.get(1002);
if (acc != nullptr) {
cout << "Account Number: " << acc->getAccountNumber() << endl;
cout << "Owner Name: " << acc->getOwnerName() << endl;
cout << "Balance: " << acc->getBalance() << endl;
}
// 更新账户余额
Account* account4 = new Account(1002, "Bob", 5000);
list.update(account4);
// 删除账户
list.remove(1003);
// 释放内存
delete account1;
delete account2;
delete account3;
delete account4;
return 0;
}
```
这是一个简单的C++实现的ATM系统,并使用链表来实现增删改查功能。
阅读全文
相关推荐
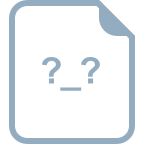
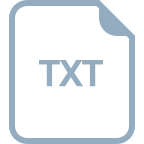
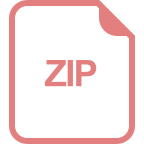
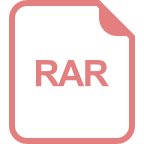
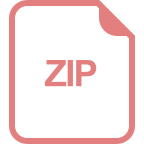
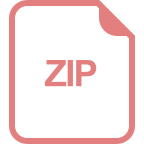
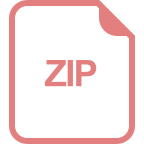
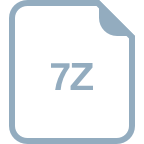
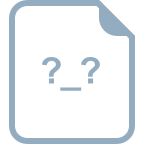
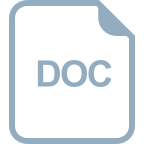
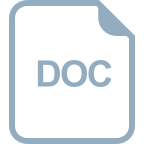