输入一个字符串,统计其空格的数量。 要求编写函数 int count_sp(const char *s); 统计并返回字符串 s 中空格的数量。 输入格式: 在一行中输入一个长度不超过 80 的字符串,其中可能含有多个空格。 输出格式: 输出共 2 行:第一行打印输入的原字符串,第二行是该字符串中空格的数量。 输入样例: 在这里给出一组输入。例如: Hello world 输出样例: 在这里给出相应的输出。例如: Hello world 1 程序样例 #include<stdio.h> #define STRLEN 80 // 返回字符串 s 中空格的数量 int count_sp(const char *s); int main (void) { char s[STRLEN + 1]; // 输入字符串 gets(s); // 输出字符串及其空格数 printf("%s\n%d\n", s, count_sp(s)); return 0; } /*** 在此实现 count_sp 函数 ***/
时间: 2024-03-16 10:47:08 浏览: 110
好的,根据你的要求,我来实现一下 `int count_sp(const char *s)` 函数,代码如下:
```c
#include <stdio.h>
#include <string.h>
#define STRLEN 80
int count_sp(const char *s);
int main() {
char s[STRLEN + 1];
gets(s);
printf("%s\n%d\n", s, count_sp(s));
return 0;
}
int count_sp(const char *s) {
int count = 0;
while (*s) {
if (*s == ' ') {
count++;
}
s++;
}
return count;
}
```
函数实现和上一题一样,只是加了一个主函数和输入输出操作。需要注意的是,`gets()` 函数已经被标记为不安全的函数,可能会存在缓冲区溢出的风险。为了避免这个问题,可以使用更安全的 `fgets()` 函数进行输入操作,如下:
```c
fgets(s, STRLEN + 1, stdin);
```
这样就可以避免缓冲区溢出的风险了。
相关问题
输入一个字符串,统计其空格的数量。 要求编写函数 int count_sp(const char *s); 统计并返回字符串 s 中空格的数量。
好的,我知道了。以下是函数 int count_sp(const char *s) 的实现:
```c
int count_sp(const char *s) {
int count = 0;
while (*s) {
if (*s == ' ') {
count++;
}
s++;
}
return count;
}
```
函数中使用了一个 while 循环,遍历字符串 s 的每一个字符。在循环中,如果当前字符是空格,就将计数器 count 加一。最后返回计数器的值即可。
希望这个函数能够满足你的需求!
输入一个字符串,统计其空格的数量。 要求编写函数 int count_sp(const char *s); 统计并返回字符串 s 中空格的数量。 输入格式: 在一行中输入一个长度不超过 80 的字符串,其中可能含有多个空格。 输出格式: 输出共 2 行:第一行打印输入的原字符串,第二行是该字符串中空格的数量。
好的,我知道了。下面是你要的函数实现:
```c
#include <stdio.h>
int count_sp(const char *s) {
int count = 0;
while (*s != '\0') {
if (*s == ' ') {
count++;
}
s++;
}
return count;
}
int main() {
char s[81];
fgets(s, 81, stdin);
printf("%s", s);
printf("%d\n", count_sp(s));
return 0;
}
```
你可以在主函数中调用 `count_sp` 函数来获取输入字符串中的空格数量。注意,该函数使用指针来遍历字符串,每当遇到空格时就将计数器加一。
相关推荐
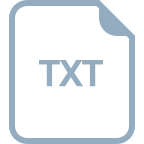
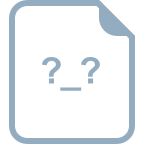
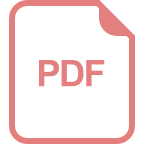








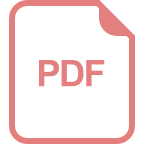
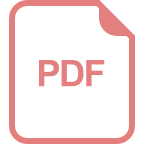
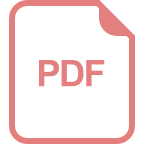
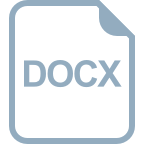