帮我写一个Django和JavaScript的文件上传下载代码,包括上传失败的处理,不使用Django内置的fileForm表单
时间: 2023-06-11 11:05:17 浏览: 104
好的,以下是一个简单的Django和JavaScript文件上传下载代码,能够处理文件上传失败的情况,不使用Django内置的fileForm表单:
在urls.py中添加以下代码:
```python
from django.urls import path
from . import views
urlpatterns = [
path('upload/', views.upload, name='upload'),
path('download/<int:id>/', views.download, name='download'),
]
```
在views.py中添加以下代码:
```python
from django.shortcuts import render, get_object_or_404
from django.http import HttpResponse, JsonResponse
from django.views.decorators.csrf import csrf_exempt
from .models import File
def upload(request):
if request.method == 'POST':
file = request.FILES.get('file')
if not file:
return JsonResponse({'status': 'error', 'msg': 'Please select a file to upload.'})
name = file.name
size = file.size
# You can do some additional checks here, such as file type and file size limit.
# ...
new_file = File(name=name, size=size)
new_file.save()
new_file.file = file
new_file.save()
return JsonResponse({'status': 'success', 'msg': 'File uploaded successfully.'})
return render(request, 'upload.html')
def download(request, id):
file = get_object_or_404(File, id=id)
response = HttpResponse(file.file, content_type='application/octet-stream')
response['Content-Disposition'] = 'attachment; filename="%s"' % file.name
return response
```
在models.py中添加以下代码:
```python
from django.db import models
class File(models.Model):
name = models.CharField(max_length=256)
size = models.IntegerField()
file = models.FileField(upload_to='uploads/')
```
在upload.html中添加以下代码:
```html
<form id="uploadForm" enctype="multipart/form-data" method="post" action="{% url 'upload' %}">
{% csrf_token %}
<input type="file" name="file" required>
<button type="submit">Upload</button>
</form>
```
在JavaScript中添加以下代码,使用jQuery库简化操作:
```javascript
$(document).ready(function() {
$('#uploadForm').submit(function(event) {
event.preventDefault();
var formData = new FormData(this);
$.ajax({
url: $(this).attr('action'),
type: 'POST',
data: formData,
success: function(response) {
alert(response.msg);
},
error: function(xhr, status, error) {
alert('An error occurred during file upload:\n' + error);
},
cache: false,
contentType: false,
processData: false
});
});
});
```
此代码中,上传的文件会保存在/media/uploads/目录下,你需要确保该目录已经存在并且可写。如果需要修改上传路径,可以在File类中的file字段的upload_to参数中定义。
阅读全文
相关推荐
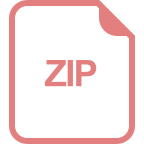
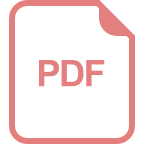
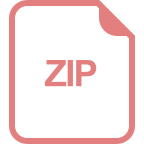
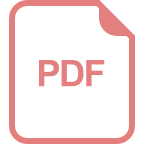
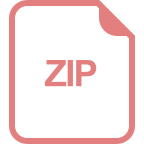
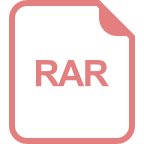
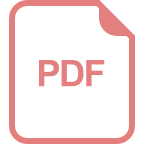
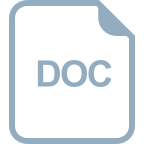
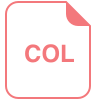
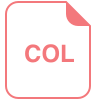
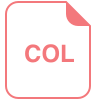
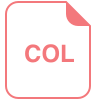
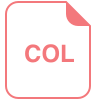
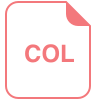
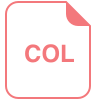
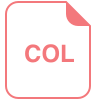
