我用的是vm模板,你用mybatisplus3.5.1帮我写一个代码生成器 ,
时间: 2024-03-11 18:51:28 浏览: 159
好的,首先需要在pom.xml中添加MyBatis Plus的依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus</artifactId>
<version>3.5.1</version>
</dependency>
```
然后,在src/main/java下创建com.example.codegenerator包,在该包下创建CodeGenerator类,用于生成代码:
```java
package com.example.codegenerator;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.*;
import com.baomidou.mybatisplus.generator.config.builder.ConfigBuilder;
import com.baomidou.mybatisplus.generator.config.po.TableFill;
import com.baomidou.mybatisplus.generator.config.po.TableInfo;
import com.baomidou.mybatisplus.generator.config.rules.DateType;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
import java.util.ArrayList;
import java.util.List;
public class CodeGenerator {
public static void main(String[] args) {
// 代码生成器
AutoGenerator mpg = new AutoGenerator();
// 全局配置
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
gc.setOutputDir(projectPath + "/src/main/java");
gc.setAuthor("Your Name");
gc.setOpen(false);
gc.setFileOverride(false);
gc.setServiceName("%sService");
gc.setIdType(IdType.AUTO);
gc.setDateType(DateType.ONLY_DATE);
gc.setSwagger2(true);
mpg.setGlobalConfig(gc);
// 数据源配置
DataSourceConfig dsc = new DataSourceConfig();
dsc.setUrl("jdbc:mysql://localhost:3306/mybatis_plus?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8");
dsc.setDriverName("com.mysql.cj.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("password");
dsc.setDbType(DbType.MYSQL);
mpg.setDataSource(dsc);
// 包配置
PackageConfig pc = new PackageConfig();
pc.setParent("com.example");
pc.setEntity("entity");
pc.setMapper("mapper");
pc.setService("service");
pc.setServiceImpl("service.impl");
pc.setController("controller");
mpg.setPackageInfo(pc);
// 策略配置
StrategyConfig strategy = new StrategyConfig();
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
strategy.setEntityLombokModel(true);
strategy.setRestControllerStyle(true);
strategy.setChainModel(true);
strategy.setLogicDeleteFieldName("deleted");
strategy.setEntityBooleanColumnRemoveIsPrefix(true);
strategy.setVersionFieldName("version");
strategy.setTableFillList(getTableFillList());
strategy.setSuperEntityClass("com.baomidou.mybatisplus.extension.activerecord.Model");
strategy.setSuperEntityColumns("id", "create_time", "update_time", "version");
strategy.setSuperServiceClass("com.baomidou.mybatisplus.extension.service.IService");
strategy.setSuperServiceImplClass("com.baomidou.mybatisplus.extension.service.impl.ServiceImpl");
strategy.setSuperMapperClass("com.baomidou.mybatisplus.core.mapper.BaseMapper");
strategy.setInclude("user");
mpg.setStrategy(strategy);
// 把配置全部注入到ConfigBuilder中
ConfigBuilder configBuilder = new ConfigBuilder(pc, dsc, strategy, new TemplateConfig(), gc);
// 获取所有表的信息
List<TableInfo> tableInfoList = configBuilder.getTableInfoList();
// 循环生成代码
for (TableInfo tableInfo : tableInfoList) {
mpg.execute(new InjectionConfig() {
@Override
public void initMap() {
// to do nothing
}
}.setFileOutConfigList(getFileOutConfigList(tableInfo, pc.getModuleName())));
}
}
private static List<TableFill> getTableFillList() {
List<TableFill> tableFillList = new ArrayList<>();
tableFillList.add(new TableFill("create_time", FieldFill.INSERT));
tableFillList.add(new TableFill("update_time", FieldFill.INSERT_UPDATE));
return tableFillList;
}
private static List<FileOutConfig> getFileOutConfigList(TableInfo tableInfo, String moduleName) {
List<FileOutConfig> fileOutConfigList = new ArrayList<>();
String entityName = tableInfo.getEntityName();
String packageName = "com.example." + moduleName;
String entityPath = "/src/main/java/" + packageName.replaceAll("\\.", "/") + "/entity/" + entityName + ".java";
String mapperPath = "/src/main/java/" + packageName.replaceAll("\\.", "/") + "/mapper/" + entityName + "Mapper.java";
String xmlPath = "/src/main/resources/mapper/" + entityName + "Mapper.xml";
String servicePath = "/src/main/java/" + packageName.replaceAll("\\.", "/") + "/service/I" + entityName + "Service.java";
String serviceImplPath = "/src/main/java/" + packageName.replaceAll("\\.", "/") + "/service/impl/" + entityName + "ServiceImpl.java";
String controllerPath = "/src/main/java/" + packageName.replaceAll("\\.", "/") + "/controller/" + entityName + "Controller.java";
fileOutConfigList.add(new FileOutConfig("/templates/entity.java.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + entityPath;
}
});
fileOutConfigList.add(new FileOutConfig("/templates/mapper.java.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + mapperPath;
}
});
fileOutConfigList.add(new FileOutConfig("/templates/mapper.xml.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + xmlPath;
}
});
fileOutConfigList.add(new FileOutConfig("/templates/service.java.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + servicePath;
}
});
fileOutConfigList.add(new FileOutConfig("/templates/serviceImpl.java.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + serviceImplPath;
}
});
fileOutConfigList.add(new FileOutConfig("/templates/controller.java.ftl") {
@Override
public String outputFile(TableInfo tableInfo) {
return System.getProperty("user.dir") + controllerPath;
}
});
return fileOutConfigList;
}
}
```
在resources目录下创建/templates/entity.java.ftl、/templates/mapper.java.ftl、/templates/mapper.xml.ftl、/templates/service.java.ftl、/templates/serviceImpl.java.ftl、/templates/controller.java.ftl这六个模板文件,用于生成代码。
最后运行CodeGenerator类即可生成代码。
阅读全文
相关推荐


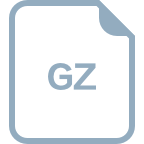















