html接收plc数据,s7-1500 连接PLC(西门子)并实现读取数据、写入数据 示例源码
时间: 2024-02-15 18:05:56 浏览: 151
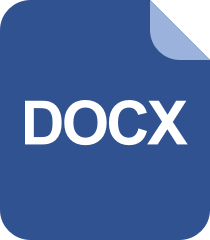
使用S7-1500的Web服务器通过本地的HTML读写PLC中的数据_示例.docx

以下是一个简单的示例代码,演示如何使用HTML通过S7-1500连接PLC,并读取和写入数据。
HTML部分:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>PLC数据读写示例</title>
</head>
<body>
<h1>PLC数据读写示例</h1>
<p>当前温度为:<span id="temp"></span></p>
<p>请输入要设置的温度:<input type="number" id="setTemp"></p>
<button onclick="setTemperature()">设置温度</button>
<script>
//读取温度数据
function getTemperature() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
document.getElementById("temp").innerHTML = xhr.responseText;
}
};
xhr.open("GET", "/temperature", true);
xhr.send();
}
//设置温度数据
function setTemperature() {
var xhr = new XMLHttpRequest();
var setTemp = document.getElementById("setTemp").value;
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
alert("设置成功!");
}
};
xhr.open("POST", "/temperature", true);
xhr.setRequestHeader("Content-Type", "application/json;charset=utf-8");
xhr.send(JSON.stringify({"setTemp": setTemp}));
}
//每隔5秒更新一次温度数据
setInterval(getTemperature, 5000);
</script>
</body>
</html>
```
JavaScript部分:
```javascript
const express = require('express');
const app = express();
const bodyParser = require('body-parser');
const S7Client = require('s7client');
//创建S7-1500连接
const s7Client = new S7Client({
name: 'PLC',
host: '192.168.0.1',
port: 102,
rack: 0,
slot: 1
});
//读取温度数据
app.get('/temperature', function(req, res) {
s7Client.read('DB1', 0, 4, function(err, data) {
if (err) {
console.error(err);
res.status(500).send('读取数据失败!');
} else {
var temperature = data.readFloatBE(0);
res.send(temperature.toFixed(1));
}
});
});
//设置温度数据
app.post('/temperature', bodyParser.json(), function(req, res) {
var setTemp = req.body.setTemp;
s7Client.write('DB1', 4, Buffer.from([setTemp]), function(err) {
if (err) {
console.error(err);
res.status(500).send('写入数据失败!');
} else {
res.send('写入数据成功!');
}
});
});
//启动服务器
app.listen(3000, function() {
console.log('服务器已启动!');
});
```
在这个示例中,我们使用了Express框架来创建一个简单的HTTP服务器,通过S7-1500连接到PLC,并实现了读取和写入数据的功能。在HTML部分,我们使用了JavaScript来定时更新温度数据,并且提供了一个输入框供用户设置温度。在JavaScript部分,我们使用了S7Client模块来连接PLC,并且使用了read和write方法来读取和写入数据。注意,在写入数据时,我们使用了body-parser模块来解析请求体中的JSON数据。
阅读全文
相关推荐
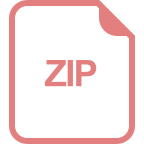
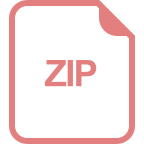
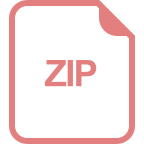
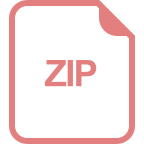
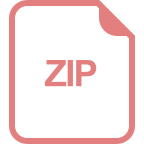
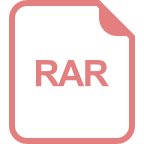
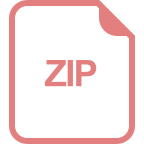
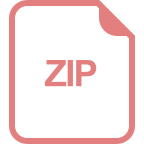
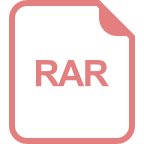
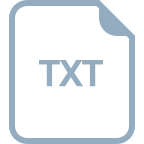
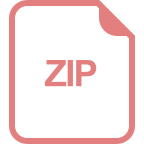
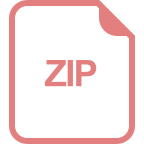
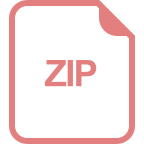
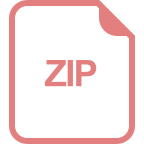
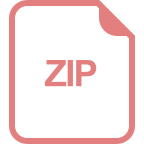
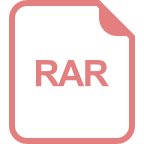
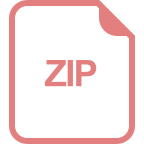